Vue Js Change SVG Size: To change the size of an SVG in Vue.js, you can use the built-in style binding to set the width and height attributes of the SVG element. This can be done by adding a style object to the SVG element, with the width and height properties set to the desired values in pixels or percentages. Alternatively, you can also use the viewBox attribute to specify the size of the SVG in relation to its content, and then use CSS to scale the SVG up or down as needed. It’s important to ensure that the aspect ratio of the SVG is maintained when resizing, to prevent distortion or stretching of the image.
Vue.js code for displaying an SVG image with a slider input for size adjustment
This is a Vue.js code snippet that displays an SVG image of an alarm clock and a slider input. The slider allows the user to adjust the size of the image by dragging the slider from left to right. The code uses Vue.js framework to bind the slider input to a size
property. The size
property updates as the user adjusts the slider.
Vue Js Change SVG SIZE Example
<div id="app" class="container">
<div class="svg-wrapper">
<svg :width="size" :height="size" xmlns="http://www.w3.org/2000/svg" fill="currentColor"
class="bi bi-alarm circle" viewBox="0 0 16 16">
<path d="M8.5 5.5a.5.5 0 0 0-1 0v3.362l-1.429 2.38a.5.5 0 1 0 .858.515l1.5-2.5A.5.5 0 0 0 8.5 9V5.5z" />
<path
d="M6.5 0a.5.5 0 0 0 0 1H7v1.07a7.001 7.001 0 0 0-3.273 12.474l-.602.602a.5.5 0 0 0 .707.708l.746-.746A6.97 6.97 0 0 0 8 16a6.97 6.97 0 0 0 3.422-.892l.746.746a.5.5 0 0 0 .707-.708l-.601-.602A7.001 7.001 0 0 0 9 2.07V1h.5a.5.5 0 0 0 0-1h-3zm1.038 3.018a6.093 6.093 0 0 1 .924 0 6 6 0 1 1-.924 0zM0 3.5c0 .753.333 1.429.86 1.887A8.035 8.035 0 0 1 4.387 1.86 2.5 2.5 0 0 0 0 3.5zM13.5 1c-.753 0-1.429.333-1.887.86a8.035 8.035 0 0 1 3.527 3.527A2.5 2.5 0 0 0 13.5 1z" />
</svg>
<input type="range" min="10" max="200" v-model="size" class="slider" style="margin-top: 20px;">
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
size: 100
}
},
});
</script>
Output of Vue Js Change SVG Size
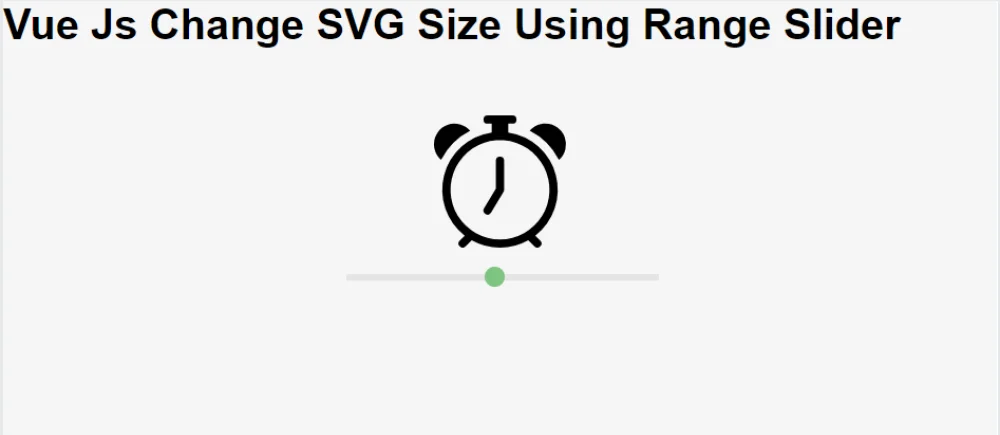