Vue.js provides a straightforward way to handle file input and, at times, it becomes crucial to validate whether the user has selected a file or left the input empty. In this tutorial, we will explore how to check if an input file is empty using Vue.js
How to Check if an Input File is Empty in Vue.js?
The HTML structure involves a simple Vue.js application with an input file element and two paragraphs that will conditionally render based on whether a file is selected or not. The critical part is the use of the @change
event listener, triggering the checkFile
method when a file is selected.
<div id="app">
<h3>Vue Js Check if Input File is Empty</h3>
<input type="file" ref="fileInput" @change="checkFile" />
<p v-if="isFileEmpty" class="empty">File is empty</p>
<p v-else class="not-empty">Selected file: {{ selectedFileName }}</p>
</div>
Here is an example of how to check if an input file is empty in Vue js
In the corresponding Vue.js script, the application instance is created, and the data
function initializes two variables: isFileEmpty
to track whether the file input is empty and selectedFileName
to store the name of the selected file.
Vue Js Check if files are selected
<script>
const app = new Vue({
el: "#app",
data() {
return {
isFileEmpty: true,
selectedFileName: ""
};
},
methods: {
checkFile() {
// Access the input element
const fileInput = this.$refs.fileInput;
// Check if files are selected
if (fileInput.files.length > 0) {
this.isFileEmpty = false;
this.selectedFileName = fileInput.files[0].name; // Display the selected file name
} else {
this.isFileEmpty = true;
this.selectedFileName = "";
}
}
}
});
</script>
The checkFile
method is crucial in determining whether the file input is empty. It begins by accessing the input element using the this.$refs.fileInput
. It then checks the length of the files
array within the input element. If there are files selected, it updates isFileEmpty
to false
and displays the name of the selected file. Otherwise, it sets isFileEmpty
to true
and clears the selectedFileName
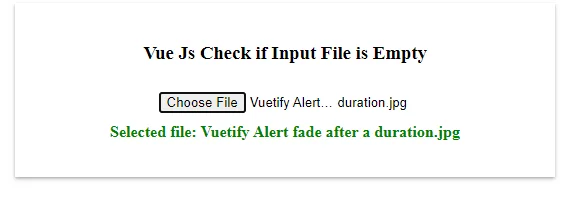
Conculsion
This approach provides a seamless way to validate file input in a Vue.js application. By leveraging the @change event and the checkFile method, developers can ensure that users receive accurate feedback based on their file selection status. This tutorial serves as a valuable resource for those looking to enhance the interactivity and user experience of their Vue.js applications involving file inputs.