Vue Js Check Whether User Device in Dark or Light Mode:To check whether the user device is in dark or light mode using Vue.js, you can utilize the matchMedia
method provided by the browser’s Window
object. This method returns a MediaQueryList
object that represents the results of a CSS media query, which can be used to detect the user’s preferred color scheme.
How can Vue Js be used to check whether a user’s device is in dark or light mode?
The Below code is a Vue.js component that checks whether the user’s device is in dark or light mode using the window.matchMedia
method, which allows you to query the user’s preferred color scheme. Here’s how the code works:
- The
data
method initializes themode
property toundefined
. - The
mounted
lifecycle hook is called after the component has been mounted on the DOM. This is where theupdateMode
method is called to set themode
property to either'dark'
or'light'
depending on whether the user’s preferred color scheme is dark or light. - The
window.matchMedia
method is used to listen for changes to the user’s preferred color scheme. When the color scheme changes, theupdateMode
method is called again to update themode
property accordingly. - The
beforeUnmount
lifecycle hook is called when the component is about to be unmounted from the DOM. This is where the event listener for color scheme changes is removed to prevent memory leaks. - The
:class
directive binds thedark-mode
orlight-mode
class to thediv
element based on the value of themode
property. - The
p
element displays whether the user’s device is in dark or light mode based on the value of themode
property.
Vue Js Check Whether User Device in Dark or Light Mode Example
<div id="app">
<div :class="mode === 'dark' ? 'dark-mode' : 'light-mode'">
<p>User Device in {{ mode === 'dark' ? 'Dark Mode' : 'Light Mode' }}</p>
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
mode: undefined,
};
},
mounted() {
this.updateMode();
window.matchMedia('(prefers-color-scheme: dark)').addEventListener('change', this.updateMode);
},
beforeUnmount() {
window.matchMedia('(prefers-color-scheme: dark)').removeEventListener('change', this.updateMode);
},
methods: {
updateMode() {
this.mode = window.matchMedia('(prefers-color-scheme: dark)').matches ? 'dark' : 'light';
},
},
})
</script>
Output of Vue Js Check Whether User Device in Dark or Light Mode
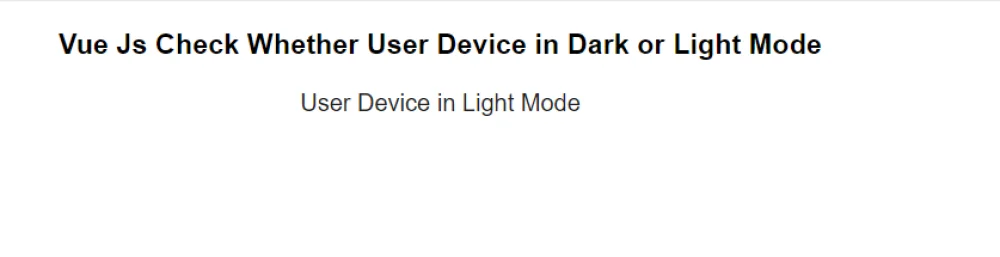