Vue Js Compare Two String: The Vue JS localecompare method provides a convenient way to compare strings and determine if they are equivalent The strings are compared based on the Unicode value of each character, and it returns a number indicating -1, 1, or 0 whether the reference string comes before, after, or is equal to the compare string in sort order. In this tutorial, we will learn how to use locale compare with Vue JS for comparing two strings.
Compare Two String in Vue JS
The first number returned by the localeCompare() method is a negative value if the reference string comes before the compare string, 0 if they are equal, and a positive value if the reference string comes after the compare string
Compare Two String in Vue JS
The first number returned by the localeCompare() method is a negative value if the reference string comes before the compare string, 0 if they are equal, and a positive value if the reference string comes after the compare string
Vue js localecompare string example
<div id="app">
<p>String 1: {{str1}}</p>
<p>String 2: {{str2}}</p>
<button @click="myFunction">click me</button>
<p>Results: {{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
str1 : 'Apple',
str2:'Banana',
results:''
}
},
methods:{
myFunction(){
this.results = this.str1.localeCompare(this.str2);
},
}
}).mount('#app')
</script>
Output of above example
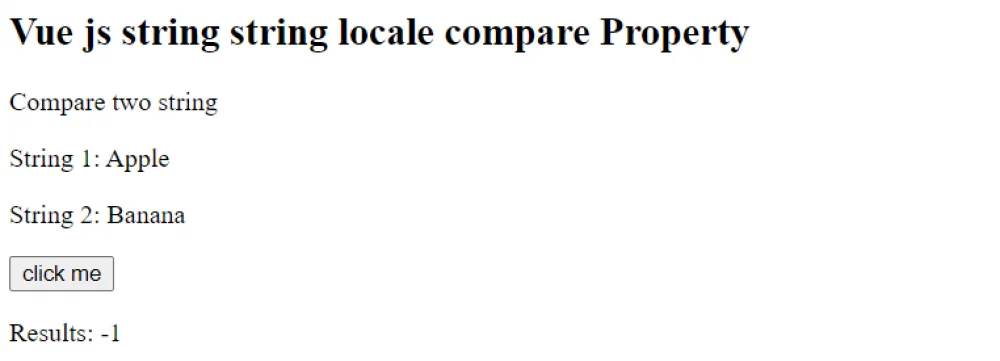
How can I compare two strings in Vue.js?
To compare two strings in Vue.js, you can use the built-in JavaScript comparison operators (==
, ===
, !=
, !==
, <
, >
, <=
, >=
) or the String methods (localeCompare()
, indexOf()
, includes()
, startsWith()
, endsWith()
, etc.) inside a computed property or a method
Vue Js Compare Two String Example
Are the strings equal? {{ areEqual }}
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
string1: 'Vue Js compare two string',
string2: 'Vue Js compare two string'
}
},
computed: {
areEqual() {
return this.string1 === this.string2;
}
}
})
</script>
Output of Vue Js Compare two string
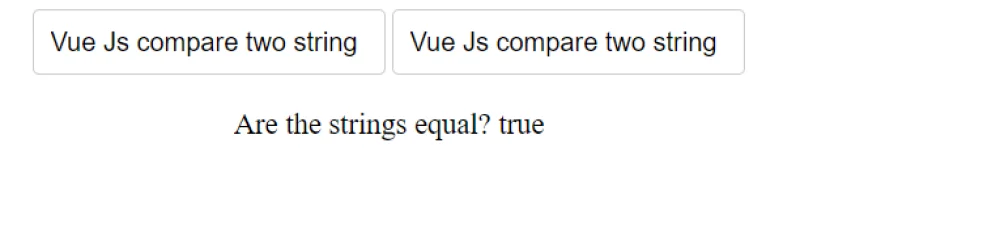