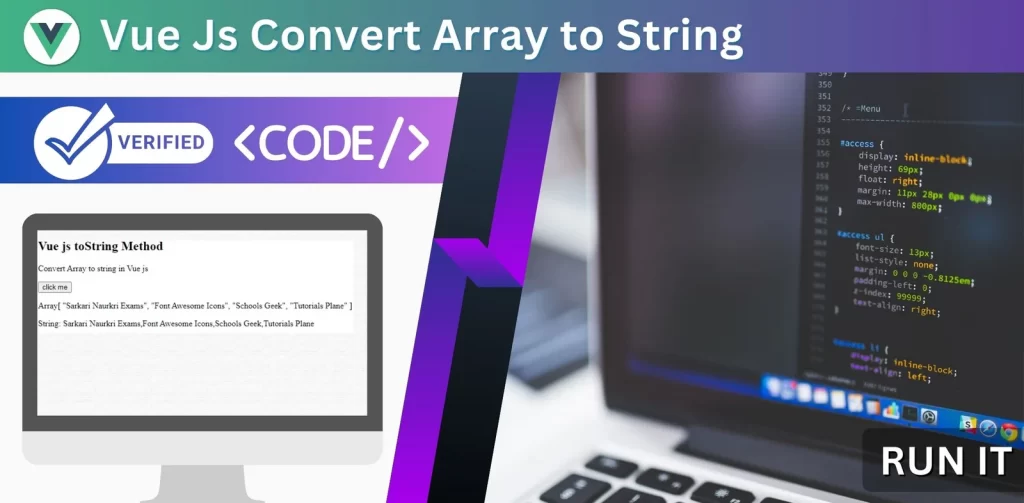
Vue Js COnvert Array to String : An array is returned as a string by the toString function. The initial array remains unchanged after using the toString function.By default, a comma (,) is used as a separator. An array can be changed into a string using the standard JavaScript toString method in Vue.js. We will describe how to convert an array into a string using the toString function in Vue.js in this article. You can modify and run the code online by using our online editor.
How to Convert Array to String in Vue Js using tostring() Javascript Method?
In Vue.js, we can easily create a stringified version of our array by applying the native javascript method function toString() to the defined array. In order to return the final string, javascript internally first turns each element into a string and then concatenates them.
Vue Js Convert Array to String Example
<div id="app">
<button @click="addFun">click me</button>
<p>Array{{dummyArray}}</p>
<p>String: {{result}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
dummyArray:['Sarkari Naurkri Exams','Font Awesome Icons','Schools Geek','Tutorials Plane'],
result:''
}
},
methods:{
addFun(){
this.result = this.dummyArray.toString();
},
}
}).mount('#app')
</script>
Output of Vue Js Convert Array to String
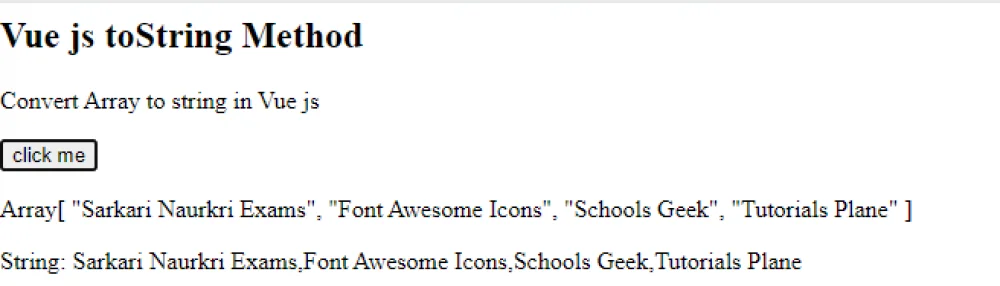
Convert JSON object to string in Vue Js
So, first, we’ll use Array.map to iterate over the object arrays and extract the title to a new array. Next, we’ll use the tostring function on the items to create the comma-separated string, as in:
Vue js JSON convert to string | Example
<div id="app">
<button @click="addFun">click me</button>
<P>Object Property:</P>
<p v-for="object in demoObject">{{object}}</p>
<p>String: {{result}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
demoObject:[{
s_no:1,
title:'Sarkari Naurkri Exams'
},
{
s_no:2,
title:'Font Awesome Icons'
},
{
s_no:3,
title:'Schools Geek'
},
{
s_no:4,
title:'Tutorials Plane'
}
],
result:''
}
},
methods:{
addFun(){
this.result = this.demoObject.map((e) =>e.title).toString();
},
}
}).mount('#app')
</script>
Output of above example
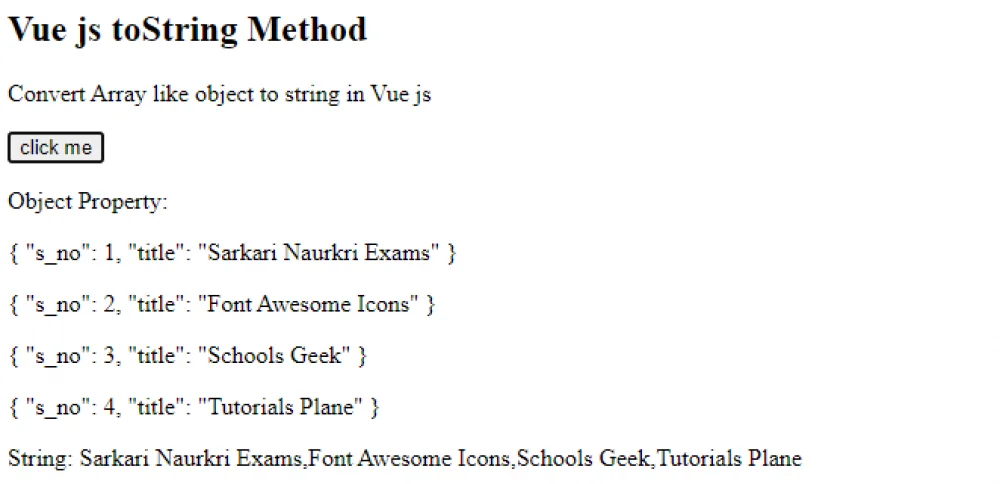