Vue Js Convert Base64 to Json Object :In Vue.js, you can convert a Base64-encoded string to a JSON object by first decoding the Base64 string and then parsing the resulting string into a JavaScript object using the JSON.parse() method. To decode the Base64 string, you can use the atob() method, which decodes a Base64 string to its original binary data.
How can I use Vue js to convert a Base64-encoded string to a JSON object?
This code uses Vue.js to convert an array of Base64 encoded strings into an array of JSON objects.
The Vue instance defines an array base64Strings
that contains two Base64 encoded strings. It also defines a jsonObjects
variable initialized to null
.
The mounted
lifecycle hook is used to call the decodeBase64
method on each element of the base64Strings
array and store the resulting JSON object in the jsonObjects
array.
The decodeBase64
method uses the atob
function to decode the Base64 string and then parses the resulting JSON string using JSON.parse
.
Vue Js Convert Base64 to Json Object Example
<div id="app">
<p>Base64 strings: {{ base64Strings }}</p>
<p>JSON objects: {{ jsonObjects }}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
base64Strings: [
'eyJpZCI6MSwiYWdlIjozMH0=',
'eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ=='
],
jsonObjects: null
};
},
mounted() {
this.jsonObjects = this.base64Strings.map(base64String => {
return this.decodeBase64(base64String);
});
},
methods: {
decodeBase64(base64String) {
const jsonString = atob(base64String);
return JSON.parse(jsonString);
}
}
})
</script>
Output of Vue Js Convert Base64 to Json Object
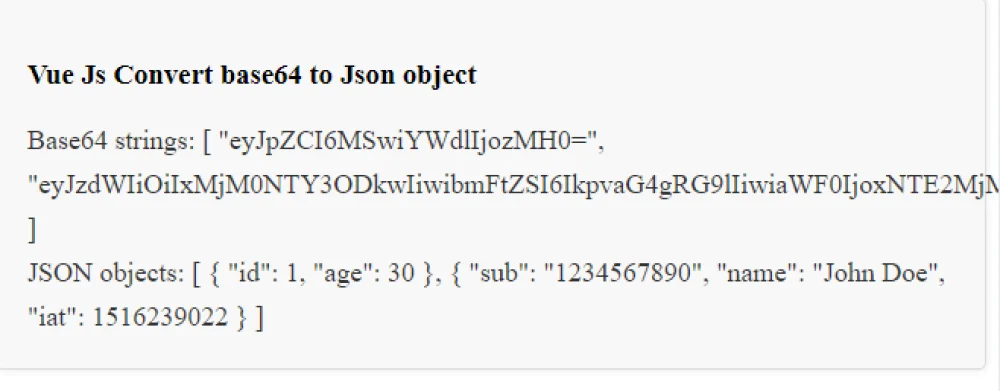