Vue Js Convert Hexa to RGB Color: we define a function called hexToRgb
that takes a hex color code as a parameter and converts it to an RGB color value. The function first removes the #
symbol from the hex color code, then extracts the red, green, and blue values from the hex code using the parseInt
function. Finally, the function returns the RGB color value as a string in the format rgb(red, green, blue)
.In this tutorial we will learn how to Convert Hexa to RGB Color or RGB Color into Hexa color in Vue Js using native javascript method.
How to Convert Hexa Color to RGB Color in Vue Js
This code defines a method called convertHexToRgb()
that takes no arguments and is a part of an object with properties hexColor
and rgbColor
.
The purpose of this method is to convert a hexadecimal color code to its corresponding RGB color value and store it in the rgbColor
property of the object.
The method first removes the “#” symbol from the hexadecimal color code by using the replace()
method.
Then it converts each of the hexadecimal values for red, green, and blue components of the color to their decimal equivalents using the parseInt()
function with a radix of 16 (since hexadecimal numbers are base-16).
It does this by extracting substrings of the hexColor string using the substring()
method, where the first parameter is the starting index and the second parameter is the ending index (not inclusive).
The first substring extracts the first two characters of the hexColor string, which represent the red component. The second substring extracts the next two characters, which represent the green component. The third substring extracts the last two characters, which represent the blue component.
Finally, it sets the rgbColor
property of the object by concatenating the values of red
, green
, and blue
variables using a template literal with the rgb()
function. The resulting string is in the format “rgb(red, green, blue)” where red
, green
, and blue
are the decimal values of the corresponding color components.
Vue Js Convert Hexa to RGB Color Example
<div id="app">
<label>Hex Color Code:</label>
<input type="text" v-model="hexColor" @input="convertHexToRgb" />
<br><br>
<label>RGB Color:</label>
<span :style="{ backgroundColor: rgbColor }"> {{ rgbColor }}</span>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
hexColor: "#ff0000",
rgbColor: "rgb(255, 0, 0)",
}
},
methods: {
convertHexToRgb() {
// remove # symbol from the hex color code
let hexColor = this.hexColor.replace("#", "");
// convert the hex color to RGB color
let red = parseInt(hexColor.substring(0, 2), 16);
let green = parseInt(hexColor.substring(2, 4), 16);
let blue = parseInt(hexColor.substring(4, 6), 16);
// set the RGB color value
this.rgbColor = `rgb(${red}, ${green}, ${blue})`;
},
},
});
app.mount('#app');
</script>
Output of Vue Js Hexa to RGB Color
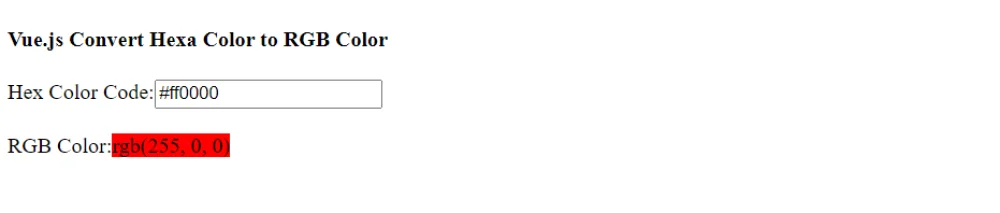
How to Convert RGB Color into Hexa Color in Vue Js
Step 1: Define the RGB color value First, you need to define the RGB color value that you want to convert to a hexadecimal color code. This can be done using an object with red
, green
, and blue
properties that represent the intensity of each color.
Step 2: Convert the RGB values to hexadecimal values Next, you need to convert each of the RGB color values to a hexadecimal value. This can be done using the toString(16)
method, which converts a decimal number to a hexadecimal string.
Step 3: Concatenate the hexadecimal values Once you have the hexadecimal values for each color, you need to concatenate them together to form a six-digit hexadecimal color code. You can do this using string concatenation or template literals
Step 4: Use the hexadecimal color code Finally, you can use the resulting hexadecimal color code in your Vue.js application to style elements with CSS or inline styles. For example, you can set the background color of an element using the background-color
property:
Vue Js RBG to Hexa Color Example
<div id="app">
<p> RGB Color : rgb({{r}}, {{g}}, {{b}})</p>
<div :style="{ backgroundColor: rgbToHex(r, g, b) }">Hexa Color: {{ rgbToHex(r, g, b) }}</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
r: 122,
g: 118,
b: 123,
}
},
methods: {
rgbToHex(r, g, b) {
let hex = ((r << 16) | (g << 8) | b).toString(16);
return "#" + "0".repeat(6 - hex.length) + hex;
}
},
});
app.mount('#app');
</script>
Output of Vue Js RGB to Hexa Color
