Vue Js string toLowerCase Method: The Vue.js framework provides a built-in method called toLowerCase() which is a useful tool for converting strings to all lowercase characters. This function can be used to ensure consistency in the formatting of strings, such as user input or data retrieved from an API. To use Vue.js’ toLowerCase() function, simply call it on a string variable or expression, and it will return a new string with all the characters converted to lowercase. This can be helpful in cases where you need to compare strings in a case-insensitive manner or display text in a uniform style. You can try out this functionality using an online Vue.js code editor for a hands-on experience.
How to Vue Js Convert string to lowercase?
This Vue.js code creates a button that, when clicked, calls the myFunction
method which converts the string FONTAWESOMEICONS
to lowercase and displays the result in the results
variable. The lowercase string is then displayed in the Lower Case
paragraph tag using Vue.js interpolation.
Here is the breakdown of the code:
- The
data
function returns an object with two properties:text
andresults
.text
is initialized with the stringFONTAWESOMEICONS
, whileresults
is initialized with an empty string. - The
myFunction
method is called when the button is clicked. It uses thetoLowerCase()
method to convert the string in thetext
property to lowercase and assigns the result to theresults
property. - The
results
property is displayed in theLower Case
paragraph tag using Vue.js interpolation ({{}}
)
Vue Js Convert String to Lowercase Example
<div id="app">
<button @click="myFunction">click me</button>
<p>Text: {{text}}</p>
<p>Lower Case :{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
text :'FONTAWESOMEICONS',
results:''
}
},
methods:{
myFunction(){
this.results = this.text.toLowerCase();
},
}
}).mount('#app')
</script>
Output of above example
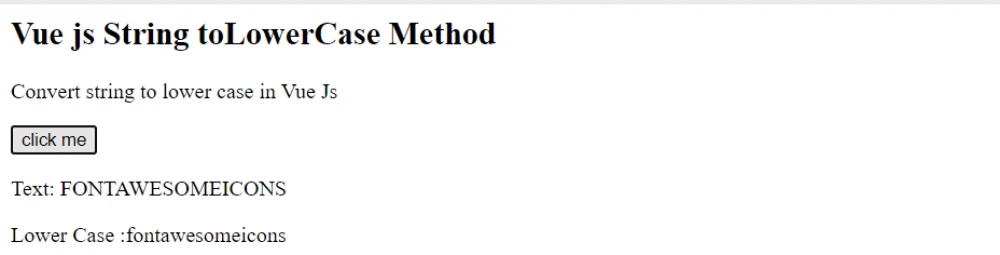
How can Vue.js automatically lowercase user input?
The built-in JavaScript toLowerCase() function in Vue.js can be used to automatically lowercase user input.
Vue Js user input lower case | Example
<div id="app">
<input type='text' placeholder='Enter Character' v-model="name" @input="myFunction"/>
<p>Lower Case :{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
name:'',
results:''
}
},
methods:{
myFunction(){
this.results = this.name.toLowerCase();
},
}
}).mount('#app')
</script>
Output of above example
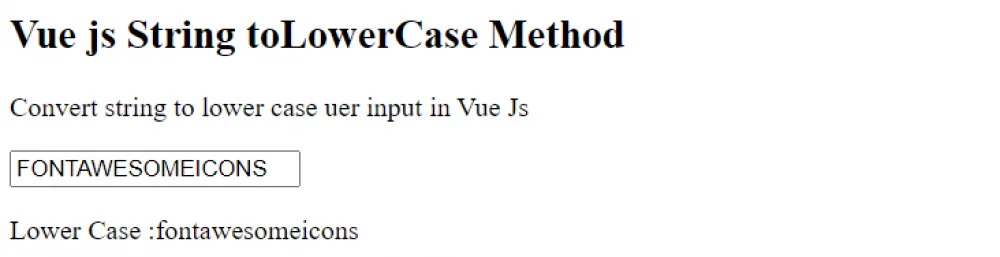