Vue Js Convert username and password to base64:Vue.js is a JavaScript framework that provides a robust set of tools for building web applications. To convert a username and password to base64 in Vue.js, you can use the built-in btoa() function. This function takes a string as an argument and returns a base64-encoded string. You can concatenate the username and password strings with a colon separator, and then pass the resulting string to the btoa() function to encode it in base64. The resulting encoded string can then be used for HTTP authentication or other purposes where base64-encoded strings are needed.
What is the syntax for converting a username and password to Base64 in Vue js?
This Vue.js code snippet will encode the provided username and password to base64 format when the submitForm()
method is called. The resulting base64 string can be used to send encoded credentials to the server. Here is a breakdown of the code:
btoa()
is a built-in JavaScript function that encodes a string in base64 format.${this.username}:${this.password}
is a string template that concatenates the username and password with a colon. For example, if the username isadmin
and the password ispassword
, this string will beadmin:password
.this.result = base64Credentials
assigns the encoded base64 string to theresult
property of the Vue instance, which can be displayed in the template.
One thing to note is that encoding the credentials in base64 format is not a secure method of sending sensitive data. It is recommended to use HTTPS and encrypted protocols for transmitting sensitive information.
Vue Js Convert username and password to base64 Example
<div id="app">
<h2>Login Form</h2>
<form @submit.prevent="submitForm">
<label for="username">Username:</label>
<input type="text" id="username" v-model="username">
<br>
<label for="password">Password:</label>
<input type="password" id="password" v-model="password">
<br>
<button type="submit">Submit</button>
<p v-if="result">Base64 string: {{result}}</p>
</form>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
username: 'admin',
password: 'password',
result: ''
};
},
methods: {
submitForm() {
const base64Credentials = btoa(`${this.username}:${this.password}`);
this.result = base64Credentials
// You can now use the base64Credentials variable to send the encoded credentials to the server
}
}
})
</script>
Output of Vue Js Convert username and password to base64
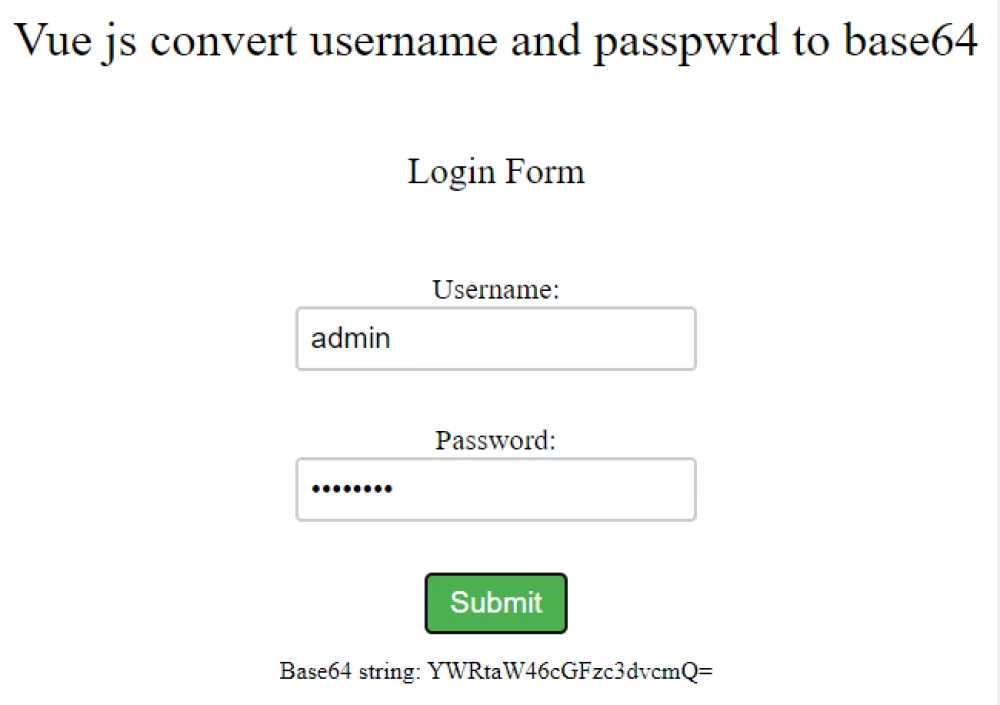