Vue Js Credit Card Number Generator:Vue.js is a popular JavaScript framework for building user interfaces. While Vue.js itself doesn’t have a built-in credit card number generator, it can be used to create a credit card number generator application. This can be achieved by creating a Vue component that utilizes JavaScript functions to generate random credit card numbers according to specific rules and validations. The component can then be integrated into a Vue.js application, allowing users to generate valid credit card numbers for testing or demonstration purposes. With Vue.js’s flexibility and event-driven architecture, creating a credit card number generator becomes achievable with relative ease.
How can I generate a credit card number using Vue.js?
This Vue.js code generates a random credit card number based on user input. The user can enter the Bank Identification Number (BIN) and the desired length of the credit card number. The code uses the Luhn algorithm to calculate the check digit, which is necessary for a valid credit card number.
When the user clicks the “Generate Credit Card” button, the code generates a random number based on the provided BIN and desired length. It then calculates the check digit using the calculateCheckDigit
method and appends it to the generated number. The final credit card number is displayed on the web page.
The code utilizes Vue.js for data binding and event handling. The v-model
directive is used to bind the user input to the corresponding data properties (bin
and length
). The @click
directive triggers the generateCreditCard
method when the button is clicked.
Vue Js Credit Card Number Generator Example
<div id="app">
<label for="bin">BIN:</label>
<input type="text" id="bin" v-model="bin" />
<br />
<label for="length">Length:</label>
<input type="number" id="length" v-model="length" />
<br />
<button @click="generateCreditCard">Generate Credit Card</button>
<p>{{ creditCardNumber }}</p>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
bin: '',
length: 16,
creditCardNumber: ''
};
},
methods: {
generateCreditCard() {
const bin = this.bin;
const length = this.length;
let generatedNumber = bin;
// Generate the remaining digits
while (generatedNumber.length < length - 1) {
generatedNumber += Math.floor(Math.random() * 10);
}
// Calculate the check digit using the Luhn algorithm
const checkDigit = this.calculateCheckDigit(generatedNumber);
// Append the check digit to the generated number
generatedNumber += checkDigit;
this.creditCardNumber = generatedNumber;
},
calculateCheckDigit(number) {
const digits = number.toString().split('').map(Number);
const checkSum = digits
.reverse()
.map((digit, index) => (index % 2 === 0 ? digit : (digit * 2 > 9 ? digit * 2 - 9 : digit * 2)))
.reduce((acc, digit) => acc + digit, 0);
const checkDigit = (10 - (checkSum % 10)) % 10;
return checkDigit.toString();
}
}
});
app.mount('#app');
</script>
Output of Vue Js Credit Card Number Generator
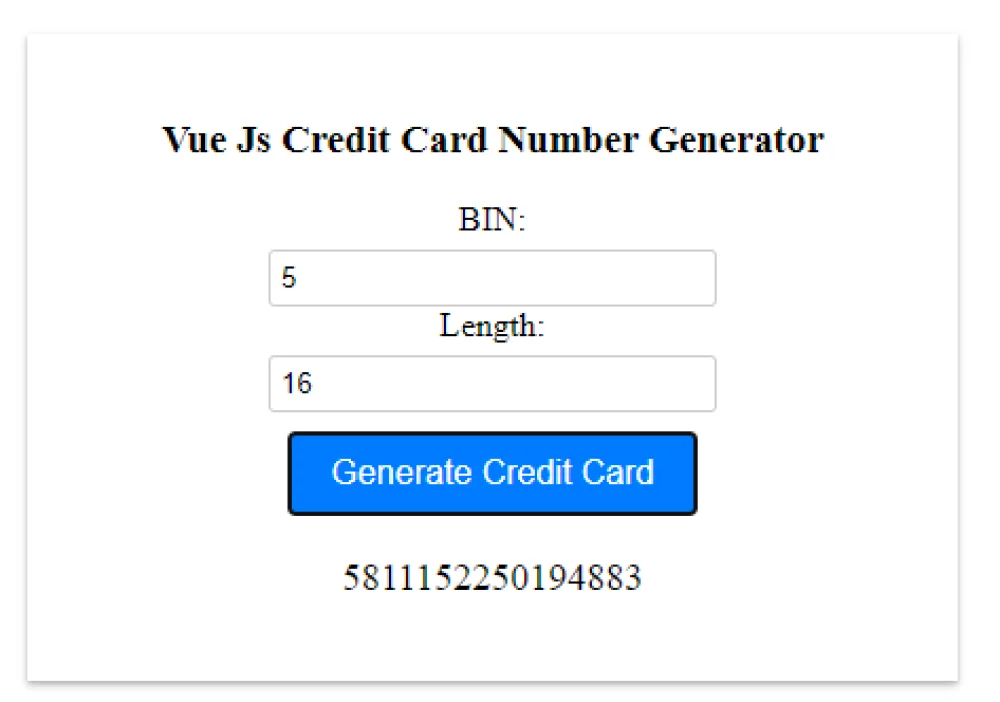