Vue Js Disable Checkbox after being checked:In Vue.js, you can disable a checkbox after it has been checked by using a combination of data binding and the :disabled
attribute. First, you need to define a boolean variable in your data section, such as isChecked
, and set it to false
. Then, in your checkbox element, bind the :disabled
attribute to the isChecked
variable using v-bind
. Lastly, add a @click
event handler to the checkbox and update the isChecked
variable to true
when the checkbox is clicked. This will disable the checkbox once it has been checked. Overall, this approach restricts further interaction with the checkbox after it is checked.
How can I disable a checkbox in Vue.js after it has been checked?
The given code snippet demonstrates how to disable a checkbox in Vue.js after it has been checked. The checkbox is bound to the isChecked
variable using v-model
. Initially, both isChecked
and isDisabled
variables are set to false
.
A watch
is set up on the isChecked
variable, so whenever its value changes, the associated callback function is triggered. In this case, when isChecked
becomes true
, the isDisabled
variable is also set to true
.
The :disabled
attribute of the checkbox is bound to the isDisabled
variable. Therefore, once the checkbox is checked and isDisabled
is true
, the checkbox becomes disabled and cannot be unchecked again.
Vue Js Disable Checkbox After Being Checked Example
<div id="app">
<input type="checkbox" id="checkbox" v-model="isChecked" :disabled="isDisabled">
<label for="checkbox">Checkbox</label>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
isChecked: false,
isDisabled: false
};
},
watch: {
isChecked(value) {
if (value) {
this.isDisabled = true;
}
}
}
});
</script>
Output of Vue Js Checkbox disable after being disabled
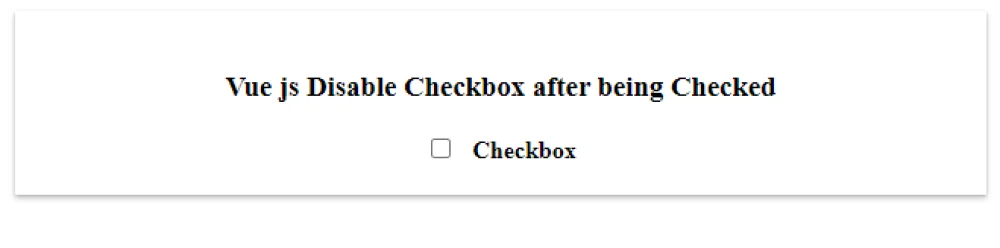
How can I disable multiple checkboxes in Vue.js after they have been checked?
The provided code is written in Vue.js and demonstrates a way to disable multiple checkboxes after they have been checked. The disabledCheckboxes
array is used to keep track of the checkboxes that should be disabled.
Each checkbox is associated with a unique identifier (checkbox1
, checkbox2
, checkbox3
). The isCheckboxDisabled
method checks if a checkbox with the given identifier exists in the disabledCheckboxes
array and returns true
if it does, indicating that the checkbox should be disabled.
The handleCheckboxChange
method is triggered when a checkbox is changed. It first checks if the checkbox is already disabled. If it is, the method returns early without making any changes. Otherwise, it disables the checkbox by adding its identifier to the disabledCheckboxes
array.
By using the :disabled
attribute binding, the checkboxes are dynamically enabled or disabled based on the values returned by the isCheckboxDisabled
method. Additionally, the disabled
class is conditionally applied to the checkbox’s label using :class="{ disabled: isCheckboxDisabled(...) }"
, providing a visual indication that the checkbox is disabled.
Vue Js Disable (Multiple) Checkbox After Being Checked Example
<div id="app">
<label class="checkbox" :class="{ disabled: isCheckboxDisabled('checkbox1') }">
<input type="checkbox" :disabled="isCheckboxDisabled('checkbox1')"
@change="handleCheckboxChange('checkbox1')" />
<span class="checkmark"></span>
Checkbox 1
</label>
<label class="checkbox" :class="{ disabled: isCheckboxDisabled('checkbox2') }">
<input type="checkbox" :disabled="isCheckboxDisabled('checkbox2')"
@change="handleCheckboxChange('checkbox2')" />
<span class="checkmark"></span>
Checkbox 2
</label>
<label class="checkbox" :class="{ disabled: isCheckboxDisabled('checkbox3') }">
<input type="checkbox" :disabled="isCheckboxDisabled('checkbox3')"
@change="handleCheckboxChange('checkbox3')" />
<span class="checkmark"></span>
Checkbox 3
</label>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
disabledCheckboxes: []
};
},
methods: {
isCheckboxDisabled(identifier) {
return this.disabledCheckboxes.includes(identifier);
},
handleCheckboxChange(identifier) {
if (this.disabledCheckboxes.includes(identifier)) {
return; // If checkbox is already disabled, do nothing
}
// Disable the checkbox and add it to the disabledCheckboxes array
this.disabledCheckboxes.push(identifier);
}
}
});
</script>
Output of Vue Js Disable Multiple Checkbox after being Checked
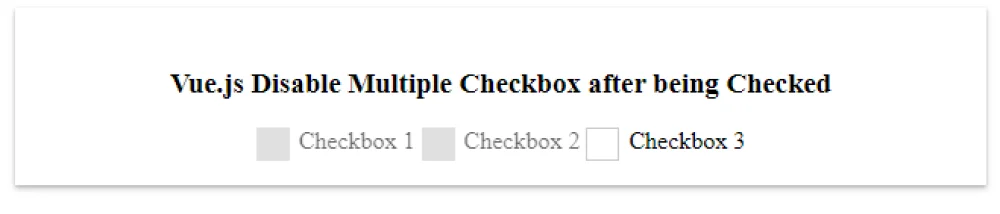