Vue js Display only first N items of list: To display only the first N items of a list in Vue.js, you can use the v-for directive along with the v-if directive. The v-for directive is used to loop through the list items, and the v-if directive is used to display only the items that meet the specified condition.
For example, if you want to display only the first three items of a list, you can use v-if=”index < 3″ inside the v-for loop. This will iterate through the list and display only the first three items that meet the condition.
This approach is useful when you have a long list of items, and you want to display only a limited number of items to improve the user experience and reduce the load time
How can I display only the first N items of a list using Vue.js?
The input element has a type attribute set to “number”, an id attribute set to “num-to-show”, and a v-model directive set to “numToShow”. The v-model directive binds the value of the input element to the “numToShow” data property in the Vue instance. The min attribute is set to 0 to prevent the user from entering negative numbers.
The unordered list contains a v-for directive that loops through each item in the “slicedItems” computed property and renders a list item for each one. The key attribute is set to “item.id” to ensure each list item is uniquely identified.
The computed property “slicedItems” returns a slice of the “developers” array. The slice starts from the beginning of the array (index 0) and ends at the index specified by the “numToShow” data property. This means that if the user enters a number in the input field, the “slicedItems” computed property will update, and the list will display only the number of items specified by the user.
Overall, this code creates a simple UI that allows the user to control the number of items displayed in a list
Vue Js Display only first N items of list Example
<div id="app">
<label for="num-to-show">Number of items to show:</label>
<input type="number" id="num-to-show" v-model="numToShow" min="0">
<ul>
<li v-for="item in slicedItems" :key="item.id">{{ item.name }}</li>
</ul>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
developers: [
{ id: 1, name: "Linus Torvalds" },
{ id: 2, name: "Tim Berners-Lee" },
{ id: 3, name: "Ada Lovelace" },
{ id: 4, name: "Grace Hopper" },
{ id: 5, name: "Alan Turing" },
{ id: 6, name: "James Gosling" },
{ id: 7, name: "Bjarne Stroustrup" },
{ id: 8, name: "Guido van Rossum" },
{ id: 9, name: "Yukihiro Matsumoto" },
{ id: 10, name: "Rasmus Lerdorf" },
{ id: 11, name: "Anders Hejlsberg" },
{ id: 12, name: "Brendan Eich" },
{ id: 13, name: "Larry Wall" },
{ id: 14, name: "Martin Odersky" },
{ id: 15, name: "John Carmack" },
],
numToShow: 3,
}
},
computed: {
slicedItems() {
return this.developers.slice(0, this.numToShow);
},
},
});
app.mount('#app');
</script>
Output of above example
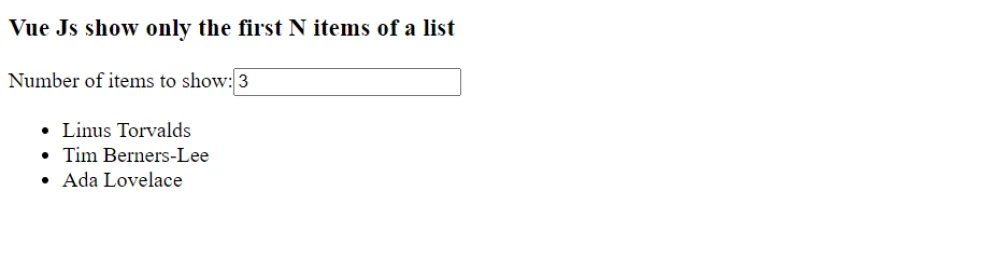