Vue Js Download Image From Url:In Vue.js, you can download an image from a URL using the built-in fetch
API. First, you would need to create a blob
object from the fetched image data. Then, you can create a URL for the blob
using the window.URL.createObjectURL()
method. Finally, you can create a new Image
object and set its src
attribute to the blob URL
, which will trigger the browser to download the image. This can be implemented in a Vue.js method or a component’s created
lifecycle hook. It is important to note that you may need to handle error cases, such as when the image URL is invalid or the network connection fails.
What is the recommended approach for downloading images from a URL using Vue js?
When the user clicks the “Download” button, the downloadImage()
method is called. This method fetches the image as a blob using fetch()
, creates a URL for the blob using URL.createObjectURL()
, creates a link element with the href
attribute set to the blob URL and the download
attribute set to the desired filename, and simulates a click on the link element using .click()
. Finally, the blob URL is revoked using URL.revokeObjectURL()
.
This approach allows the user to download the image without leaving the page or opening it in a new tab.
Vue Js Download Image From Url Example
<div id="app">
<img :src="imageUrl" />
<button @click="downloadImage">Download</button>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
imageUrl: 'https://pixabay.com/get/g6b89266906d58b0b749503048edf368407651e9c300c3a713450b22391af7a3430cb31dc7bb80495d1cb3a97bb895f94_640.jpg',
};
},
methods: {
async downloadImage() {
const blob = await (await fetch(this.imageUrl)).blob();
const url = URL.createObjectURL(blob);
Object.assign(document.createElement('a'), { href: url, download: 'image.jpg' })
.click();
URL.revokeObjectURL(url);
}
},
})
</script>
Output of Vue Js Download Image from Url
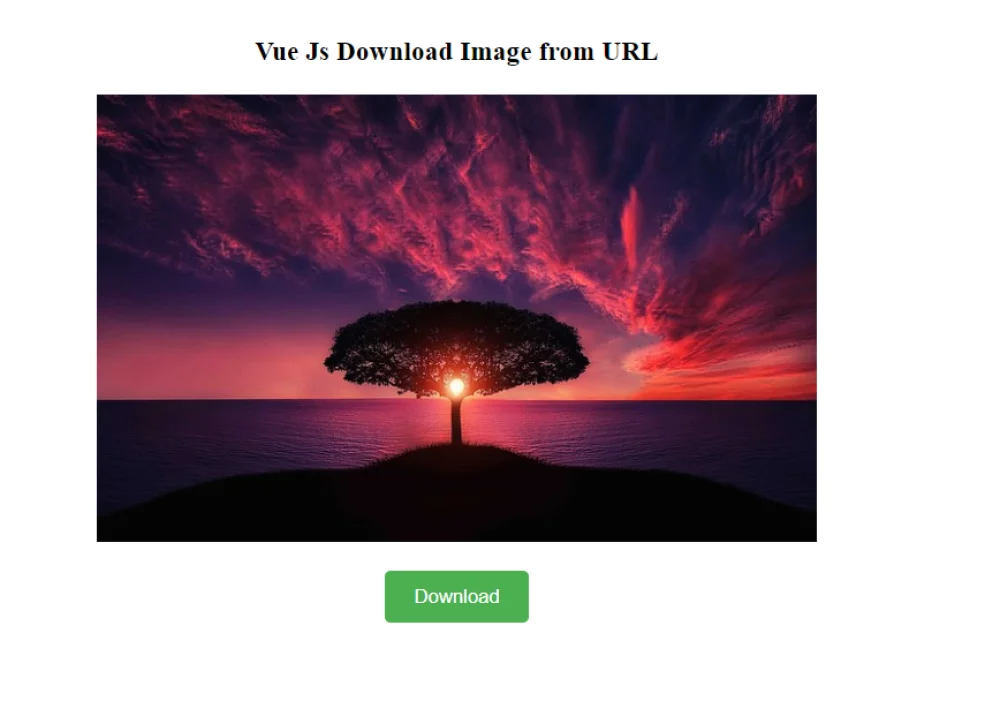