Vue Js filter by Category: It involves creating a computed property that listens for changes to both the table data and the selected category. This computed property returns a filtered array of objects that match the selected category.
Vue JS Pagination with Search: Vue.js also offers pagination functionality that allows users to navigate through pages with ease. To implement this feature, you can use Vue js Pagination with search to create a powerful data table with pagination and search functionality.
Vue Js Custom search filter with Vue Js Pagination: You can create a custom highly responsive and user-friendly data table that allows users to Vue Js filter by a category, search data, and navigate through multiple pages. Overall, Vue js pagination with search provides a flexible and dynamic way to search, filter and paginate table data.
In this tutorial we will learn how to implement vue js filter by category,pagination with search in table
How to implement filter by category and Vue JS Pagination with search in the table?
This is a Vue js filter by category application that displays a table of developers with their names, categories, and IDs. The table can be filtered by category using a dropdown menu and Search by Name. The data for the developers is stored in an array of objects called items, with each object containing an id, name, and category property. The Vue.js app is created using the Vue.createApp method. The app has a data property that contains an array of categories and the selected category. The computed property returns a filtered list of items based on the selected category. The v-for directive is used to loop through the items and display them in the table. The v-model directive is used to bind the value of the dropdown menu to the selectedCategory data property.
This is a Vue js pagination and search application that displays a custom table of developers and allows users to filter and search for specific developers. The application uses a data object to store the list of developers, as well as the selected category and search query. It also uses computed properties to filter and paginate the data based on the user’s inputs.
The HTML code contains a dropdown menu for selecting the category, an input field for searching, a table to display the data, and pagination buttons. The Vue.js app is initialized and mounted on the HTML element with the ID “app”.
The Vue Js Pagination with search code defines a Vue.js application that includes a data object, computed properties, and methods. The data object contains the list of developers, the selected category, the search query, the current page, and the number of items to display per page.
The computed properties include a filteredItems array, which filters the list by using Vue Js filter by category and custom search query, a totalPages property, which calculates the total number of pages based on the number of items per page and the number of filtered items, and a paginatedItems array, which returns a subset of the filtered items based on the current page and the number of items per page.
The Vue Js Pagination demo includes nextPage and prevPage functions, which increment and decrement the current page number, respectively, as long as the user is not on the first or last page.
Example: Vue JS Pagination & Search In table | Vue Js Filter By Category
<div id="app">
<label for="category-filter">Filter by category:</label>
<select id="category-filter" v-model="selectedCategory">
<option v-for="category in categories" :key="category">{{ category }}</option>
</select>
<label for="search-input">Search:</label>
<input type="text" id="search-input" v-model="searchQuery">
<table class="developer-table">
<thead>
<tr>
<th>Id</th>
<th>Developer Name</th>
<th>Category</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in paginatedItems" :key="item.id">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.category }}</td>
</tr>
</tbody>
</table>
<div class="pagination">
<button :disabled="currentPage === 1" @click="prevPage">Previous</button>
<span>{{ currentPage }} / {{ totalPages }}</span>
<button :disabled="currentPage === totalPages" @click="nextPage">Next</button>
</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
categories: ["All", "Frontend Developer", "Backend Developer", "Full Stack Developer"],
selectedCategory: "All",
searchQuery: "",
items: [
{ id: 1, name: "John Smith", category: "Frontend Developer" },
{ id: 2, name: "Sarah Johnson", category: "Backend Developer" },
{ id: 3, name: "David Chen", category: "Full Stack Developer" },
{ id: 4, name: "Emily Lee", category: "Backend Developer" },
{ id: 5, name: "Michael Wong", category: "Full Stack Developer" },
{ id: 6, name: "Rachel Patel", category: "Frontend Developer" },
{ id: 7, name: "Kevin Davis", category: "Frontend Developer" },
{ id: 8, name: "Jennifer Kim", category: "Backend Developer" },
{ id: 9, name: "James Garcia", category: "Full Stack Developer" },
{ id: 10, name: "Laura Nguyen", category: "Backend Developer" },
{ id: 11, name: "Brandon Brown", category: "Full Stack Developer" },
{ id: 12, name: "Samantha Taylor", category: "Frontend Developer" },
],
currentPage: 1,
itemsPerPage: 3,
};
},
computed: {
filteredItems() {
let filteredItems = this.items;
if (this.selectedCategory !== "All") {
filteredItems = filteredItems.filter(item => item.category === this.selectedCategory);
}
if (this.searchQuery !== "") {
filteredItems = filteredItems.filter(item => item.name.toLowerCase().includes(this.searchQuery.toLowerCase()));
}
return filteredItems;
},
totalPages() {
return Math.ceil(this.filteredItems.length / this.itemsPerPage);
},
paginatedItems() {
const startIndex = (this.currentPage - 1) * this.itemsPerPage;
return this.filteredItems.slice(startIndex, startIndex + this.itemsPerPage);
},
},
methods: {
nextPage() {
if (this.currentPage < this.totalPages) {
this.currentPage++;
}
},
prevPage() {
if (this.currentPage > 1) {
this.currentPage--;
}
},
},
});
app.mount('#app');
</script>
Output of Vue Js Table FIlter by Category and Search
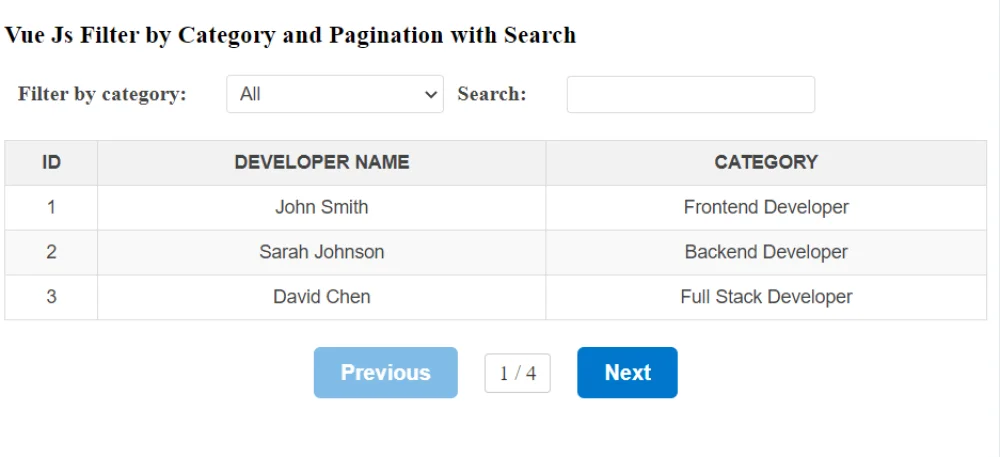