Vue Js Generate Secret Key | Private Key:In Vue.js, you can generate a secret key using the native JavaScript method crypto.getRandomValues()
. This method is designed to generate cryptographically secure random values, making it ideal for generating secret keys. By utilizing this method, you can ensure that the secret key is truly random and cannot be easily guessed or reproduced by an attacker. It’s important to note that this method is only available in modern
browsers that support the Web Cryptography
API. By using this method, you can generate a secure and robust secret key for your Vue.js application.
What is the process for generating a secret key or private key in Vue js?
The code generates a secret key using the crypto.getRandomValues()
function, encodes it as a base64 string using the btoa()
function, and sets it as the secretKey
property in the Vue instance’s data
object.
To use this code, you can include it in your Vue.js application’s HTML file, between the <script>
tags. You can then call the generateSecretKey()
method in your Vue instance wherever you need to generate a secret key.
Vue Js Generate Secret Key | Private Key Example
<div id="app">
<button @click="generateSecretKey">Generate Secret Key</button>
<p v-if="secretKey">Secret Key: {{ secretKey }}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
secretKey: null,
};
},
methods: {
generateSecretKey() {
// Generate a secret key using crypto.getRandomValues()
let secretKey = new Uint8Array(32); // 32 bytes = 256 bits
window.crypto.getRandomValues(secretKey);
// Convert the secret key to a base64-encoded string
let base64SecretKey = btoa(String.fromCharCode.apply(null, secretKey));
// Set the secret key in the component's data
this.secretKey = base64SecretKey;
},
},
})
</script>
Output of Vue Js Generate Secret Key | Private Key
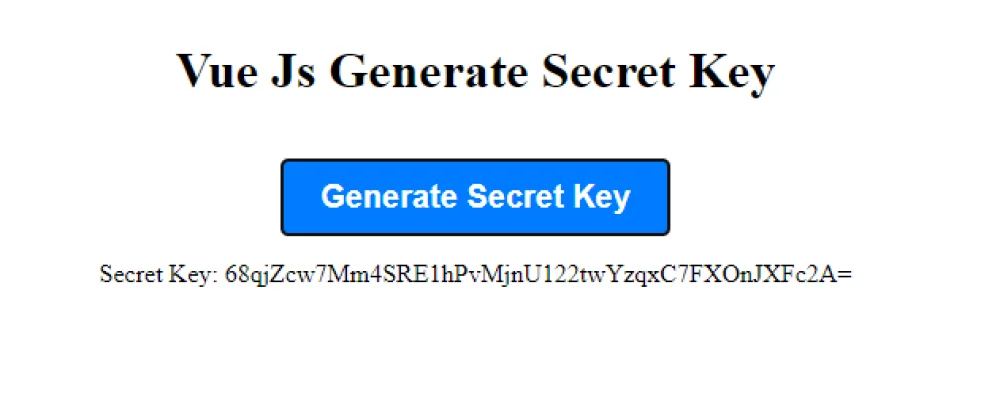