Vue Js Find Minimum value from Array: Vue.js makes it simple to find the minimum value in an array. This can be achieved by using the built-in Math.min() method, which takes an array of numbers as its argument and returns the minimum value within that array.These tutorials will teach you how to use native JavaScript and Vue.js to find the minimum value in an array or an array of objects.
Find the minimum element of an array in Vue Js?
In Vue.js, the minimum element of an array can be found by using the Math.min() method.which takes an array as an argument and returns the minimum value in that array.Return the minimum value of the array in the example below.
Vue Js Min Value of Array | Example
<div id="app">
<button @click="getMinValue">click me</button>
<p>{{minValue}}</p>
</div>
<script type="module">
import { createApp } from "vue";
createApp({
data() {
return {
array: [2, 5, 3, 1, 6, 9, 5],
minValue: ''
}
},
methods: {
getMinValue() {
this.minValue = Math.min.apply(Math, this.array);;
}
}
}).mount("#app");
</script>
Output of above example
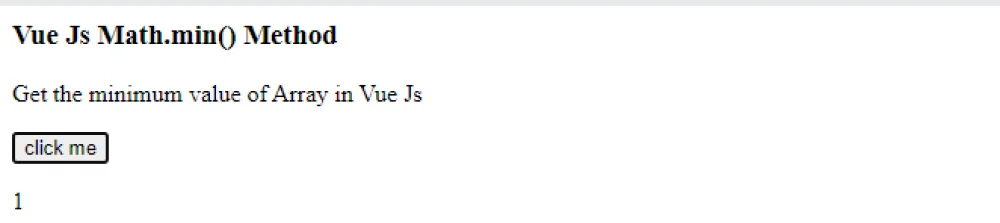
Vue Js Get Minimum Value using Spread operator| Example
this.minValue = Math.min(...this.array)
How to find the minimum value of a property in an array object?
To get the minimum value of a property in an array of objects, use the Math.min() method in combination with the map() method to extract the minimum property value of the array of objects. You can get the minimum value from an array in VueJS simply as follows:
Vue Js Min Value Array Object | Example
<div id="app">
<button @click="getMinValue">click me</button>
<p>{{minPrice}}</p>
</div>
<script type="module">
import { createApp } from "vue";
createApp({
data() {
return {
arrayObj: [
{ fruit: 'Apple', price: 100 },
{ fruit: 'Mango', price: 50 },
{ fruit: 'Orange', price: 70 },
{ fruit: 'Pineapple', price: 120 },
{ fruit: 'Banana', price: 20 },
],
minPrice: ''
}
},
methods: {
getMinValue() {
this.minPrice = Math.min(...this.arrayObj.map(property => property.price))
}
}
}).mount("#app");
</script>
Output of above example
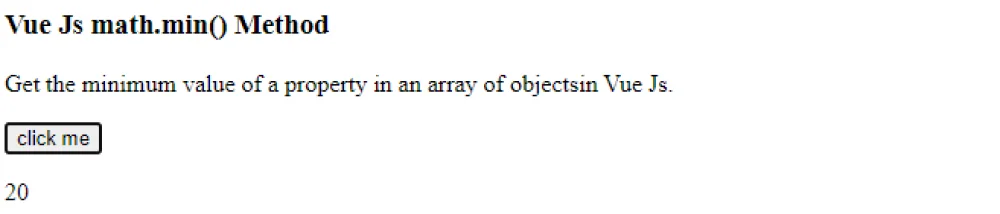