Vue Js local storage: Vue.js’s local storage provides a convenient way to store data locally, allowing web applications to access and modify the data without having to rely on a server. The localStorage API is part of the browser’s JavaScript API and is a simple key-value store. You can use local storage to set data, get data, and remove data from local storage. Using localStorage.setItem(‘key’, “value”), localStorage.getItem(‘key’), and localStorage.removeItem(‘key’), you can store data locally. In this tutorial, we will learn how to add, get, delete, and store data locally with the help of vue js and native javascript.
Vue Js Set data in local storage
In Vue.js, you can use the localStorage property of a window object to set an item in local storage by using the localStorage.setItem(‘key’,’item’) method. This will set data in local storage with the key and value.
Vue Js set value in local storage syntax
localStorage.setItem('data', "fontawesomeicons.com")
Vue Js Get data in local storage
In Vue.js, you can use the localStorage property of a window object to get an item from local storage by using the localStorage.getItem(“key”) method. This will get the data from local storage with the value
Vue Js get value in local storage syntax
var myData = localStorage.getItem('data');
Vue Js Remove data in local storage
In Vue.js, you can use the localStorage property of a window object to remove an item from local storage by using the localStorage.removeItem(“key”) method. This will delete the data from local storage
Alternatively, you can use localStorage.clear() is a useful method for removing all stored data from a browser window
Vue Js remove value in local storage syntax
localStorage.removeItem('data');
The full demonstration is given below, to get, set, and remove data from local storage.
<div id="app">
<p>{{localstoreData}}</p>
<button @click="setData">Set items to local storage</button><br><br>
<button @click="getData">Get items from local storage</button><br><br>
<button @click="removeData">Delete item from local storage</button><br><br>
</div>
<script type="module">
import { createApp } from "vue";
createApp({
data() {
return {
data: 'Apple',
localstoreData: localStorage.getItem('fruit')
}
},
methods: {
setData() {
localStorage.setItem('fruit', this.data);
alert('Sucessfully Data added into localstorage');
},
getData() {
this.localstoreData = localStorage.getItem('fruit')
},
removeData() {
localStorage.removeItem('fruit')
this.localstoreData = localStorage.getItem('fruit');;
alert('Deleted data')
}
}
}).mount("#app");
</script>
Output of above example
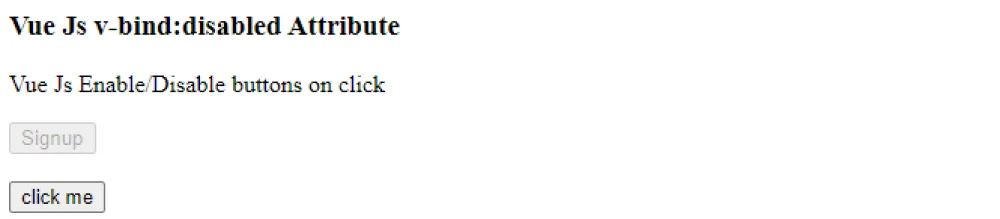