Vue Js mask a string with asterisks (*) show only last 4 digit:In Vue.js, you can mask a string by replacing all characters except the last four digits with asterisks (). This can be achieved by using a combination of string manipulation and the slice method. First, you determine the length of the string and extract the last four digits using the slice method. Then, you generate a new string by repeating the asterisk character () for the remaining characters. Finally, you concatenate the asterisk string with the last four digits to obtain the masked string.
How can I mask a string in Vue.js with asterisks (*) and display only the last 4 digits?
This Vue.js code snippet demonstrates how to mask a string with asterisks (*) and show only the last four digits. The original number is stored in the originalNumber
data property. In the computed property maskedNumber
, a check is performed to see if the length of the original number is less than or equal to four.
If it is, the original number is returned as is. Otherwise, a string of asterisks is generated using the repeat
method, based on the length of the original number minus four.
The last four digits are extracted using the slice
method. Finally, the masked digits and the last four digits are concatenated and returned as the maskedNumber
.
Vue Js mask a string with asterisks (*) show only last 4 digit
<div id="app">
<p>Original Number: {{ originalNumber }}</p>
<p>Masked Number: {{ maskedNumber }}</p>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
originalNumber: '1234567890'
};
},
computed: {
maskedNumber() {
if (this.originalNumber.length <= 4) {
return this.originalNumber;
}
const maskedDigits = '*'.repeat(this.originalNumber.length - 4);
const lastFourDigits = this.originalNumber.slice(-4);
return maskedDigits + lastFourDigits;
}
}
});
app.mount('#app');
</script>
Output of Vue Js mask a string with asterisks (*) show only last 4 digit
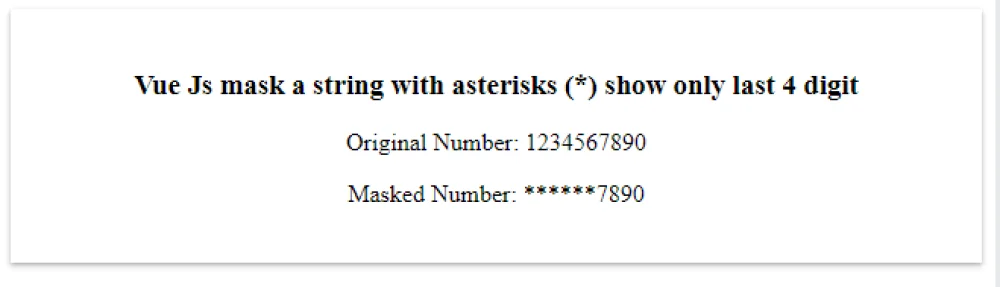