Vue Js Modal Close Button:In Vue.js, to create a close button for a modal, you can use the v-on directive to bind a click event to a method that will handle the modal’s closing logic. For example, you can add a button element with the v-on:click directive and set it to call a closeModal method. Inside the closeModal method, you can update a data property that controls the visibility of the modal by setting it to false. This will trigger the modal to close and disappear from the user interface. Overall, the close button provides an interactive way for users to dismiss the modal window in a Vue.js application.
This is a Vue.js code snippet that demonstrates a simple modal component with a close button.
When the page loads, there is a button labeled “Open Modal.” Clicking this button triggers the openModal
method, which sets the showModal
data property to true
. This property controls whether the modal is displayed.
Inside the v-if
directive, there is a div
with the modal
class that is conditionally rendered based on the value of showModal
. When the showModal
property is true
, the modal becomes visible.
Within the modal, there is a close button represented by the span
element with the class close
. Clicking on this button triggers the closeModal
method, which sets showModal
to false
, effectively hiding the modal.
The modal content is defined within the modal-content
class, including a heading and a paragraph element.
This code snippet provides a basic implementation of a modal component with the ability to open and close it using Vue.js
Vue Js Modal Close Button Example
<div id="app">
<button @click="openModal">Open Modal</button>
<div v-if="showModal" class="modal">
<div class="modal-content">
<span class="close" @click="closeModal">×</span>
<h2>Vue Js Modal Close Button</h2>
<p>Modal content goes here.</p>
</div>
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
showModal: false
};
},
methods: {
openModal() {
this.showModal = true;
},
closeModal() {
this.showModal = false;
}
}
});
</script>
Output of Vue Js Modal Close Button
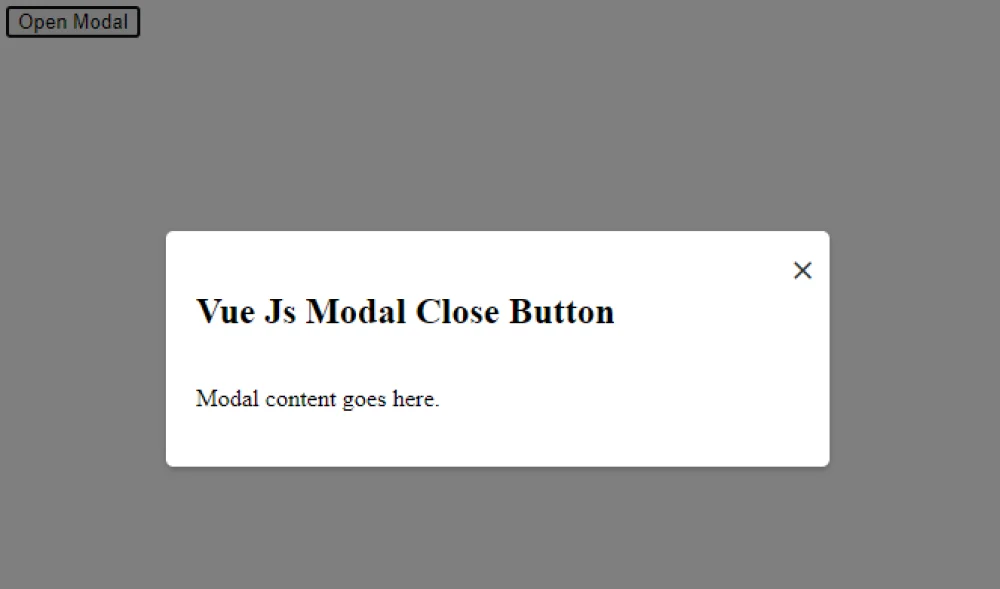
To style a Vue.js modal close button using CSS, you can target the button element within the modal component and apply custom styles using CSS properties such as background-color, font-size, padding, etc.
You can use class selectors, ID selectors, or element selectors to target the button and modify its appearance.
Vue Js Modal Close Button – CSS Code Example
.modal {
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgba(0, 0, 0, 0.5);
display: flex;
justify-content: center;
align-items: center;
}
.modal-content {
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.2);
width: 400px;
display: flex;
flex-direction: column;
position: relative;
}
.close {
color: #333333;
position: absolute;
top: 10px;
right: 10px;
cursor: pointer;
font-size: 28px;
font-weight: bold;
transition: color 0.3s ease;
}
.close:hover,
.close:focus {
color: #FF0000;
/* Bright red color on hover and focus */
text-decoration: none;
}