Vue Js Open Close Sidebar: To achieve Vue Js open close sidebar functionality using CSS transformations like translateX()
, you can follow these steps. First, define a data property called sidebarOpen
and set it to false
. Next, create a method called toggleSidebar
that toggles the value of sidebarOpen
between true
and false
. In your template, use a v-bind
directive to bind the class of the sidebar element. For example, <div :class="{ 'sidebar-open': sidebarOpen }">
. In your CSS, define the .sidebar-open
class with the desired CSS transformations, such as transform: translateX(0);
to open the sidebar or transform: translateX(-100%);
to close it. Finally, add a button or an element that triggers the toggleSidebar
method on click. When clicked, it will toggle the sidebarOpen
property, applying the appropriate class and animating the sidebar’s opening and closing using the CSS transformations.
How to Create Vue Js Open Close Sidebar Menu?
This code snippet that demonstrates how to implement an Vue Js open close sidebar functionality. The code consists of a container with a toggle button, a sidebar, and a main content section.
When the toggle button is clicked, it triggers the toggleSidebar
method. The sidebar’s visibility is controlled using the isOpen
property, which determines whether the sidebar should have the class “sidebar-open” applied to it.
Clicking the close button within the sidebar also triggers the toggleSidebar
method. The sidebar content includes a simple unordered list menu.
Vue Js Open Close Sidebar – Html Code
<div id="app">
<div class="container">
<button class="toggle-button" @click="toggleSidebar">
<i class="fas" :class="isOpen ? 'fa-times' : 'fa-bars'"></i>
</button>
<div class="sidebar" :class="{ 'sidebar-open': isOpen }">
<button class="close-button" @click="toggleSidebar">
<i class="fas fa-times"></i>
</button>
<!-- Sidebar content -->
<ul>
<li>Menu Item 1</li>
<li>Menu Item 2</li>
<li>Menu Item 3</li>
</ul>
</div>
<div class="main-content">
<!-- Main content -->
<h1>Vue Js Open Close Sidebar | Toggle Sidebar</h1>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p>
</div>
</div>
</div>
Output of Vue Js Open Close Sidebar
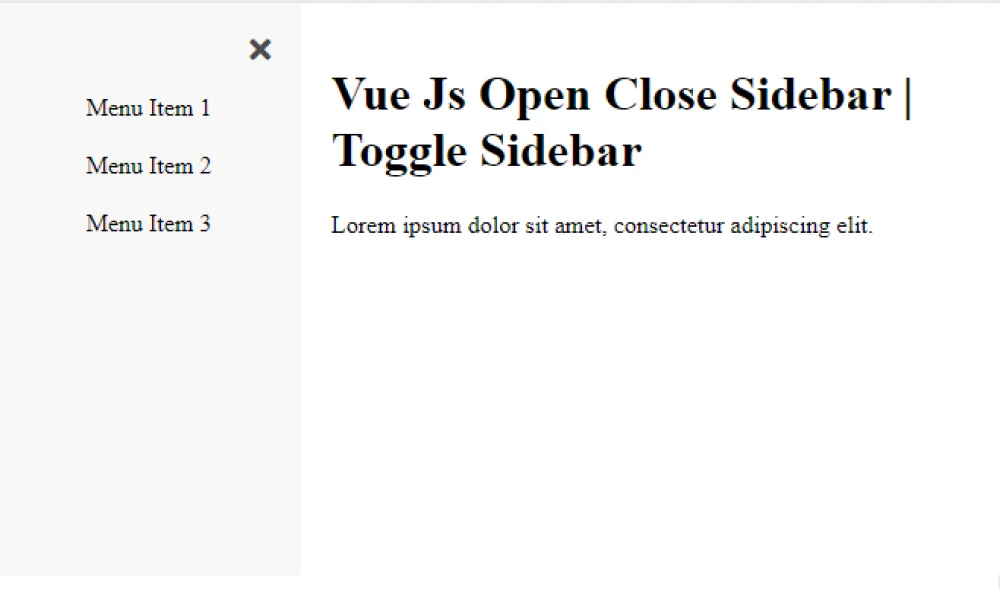
Vue JS Open Close Sidebar with Javascript Code
This script uses Vue.js to create an application with a data property called “isOpen” that represents the state of the sidebar. Initially, the sidebar is set to be closed.
The script also includes a method called “toggleSidebar” which toggles the value of “isOpen” when called. When the app is mounted, it is associated with the element with the id “app”. This allows the Vue js open close sidebar.
Vue Js Open Close Sidebar – Javascript Code
<script>
const app = Vue.createApp({
data() {
return {
isOpen: true, // Initially sidebar is closed
};
},
methods: {
toggleSidebar() {
this.isOpen = !this.isOpen; // Toggle the value of isOpen
},
},
});
app.mount('#app');
</script>
Vue JS Open Close Sidebar with CSS
This CSS code defines a sidebar layout in Vue.js. The sidebar is initially hidden off-screen using the transform: translateX(-100%);
property.
When the sidebar is toggled, the sidebar-open
class is applied, which sets the transform
property to translateX(0)
and slides the sidebar into view.
The sidebar contains a toggle button and a close button positioned at the top corners. The main content area occupies the remaining space.
Vue Js Open Close Sidebar – Css Code
body {
margin: 0;
}
.container {
display: flex;
height: 100vh;
}
.sidebar {
width: 200px;
background-color: #f8f8f8;
transition: transform 0.3s ease-in-out;
transform: translateX(-100%);
position: relative;
margin: 0;
}
.sidebar-open {
transform: translateX(0);
}
.main-content {
flex: 1;
padding: 20px;
background-color: #ffffff;
max-width: 1200px;
margin: 0 auto;
box-shadow: 0 1px 2px 0 rgba(0, 0, 0, 0.12), 0 2px 4px 0 rgba(0, 0, 0, 0.24);
width: 100%;
}
.toggle-button,
.close-button {
position: absolute;
top: 10px;
padding: 10px;
background-color: transparent;
color: #4a4a4a;
border: none;
border-radius: 50%;
cursor: pointer;
font-size: 20px;
transition: background-color 0.3s ease-in-out;
}
.toggle-button:hover,
.close-button:hover {
background-color: #e0e0e0;
}
.toggle-button:focus,
.close-button:focus {
outline: none;
}
.toggle-button i,
.close-button i {
font-size: inherit;
}
.toggle-button {
left: 10px;
}
.close-button {
right: 10px;
}
ul {
list-style-type: none;
padding: 0;
margin: 0;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
margin-top: 50px;
}
li {
padding: 10px;
cursor: pointer;
transition: background-color 0.3s ease-in-out;
}
li:hover {
background-color: #f8f8f8;
}
li.active {
background-color: #e0e0e0;
}
.sidebar:hover {
background-color: #e0e0e0;
}