Vue Js OTP Input: Vue Js OTP input is a customizable component that allows users to input a one-time password (OTP) in their Vue.js applications. This component provides a secure and user-friendly way to input OTPs, with features like validation and mask for the input fields. It can also handle different OTP input types, such as numeric or alphanumeric. The Vue Js OTP input component can be easily integrated into existing Vue.js applications, with options for customization to fit the app’s design and functionality. Overall, the Vue Js OTP input component is a convenient and secure way to manage OTP inputs in Vue.js applications.
Create Vue Js OTP Input Example
This Vue Js application creates a custom OTP input component that consists of four input fields, each accepting a single character. The component stores the input characters in the otpValues array, according to the corresponding index. The component also moves the focus to the next input field when a user types a character, and deletes the character in the current field when the backspace key is pressed.
The @input and @keydown.backspace directives are used to listen to user input events. In addition, if the current input field is empty and not the first one, the focus is moved to the previous field. The input fields are accessed using the ref attribute in the component.
Vue Js OTP Input Example
<div id="app">
<div class="otp-container">
<input v-for="(value, index) in otpValues" :key="index" type="text" maxlength="1" class="otp-input"
:value="value" @input="handleInput(index, $event.target.value)"
@keydown.backspace="handleBackspace(index)" ref="otpFields" />
</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
otpValues: ['', '', '', ''],
}
},
methods: {
handleInput(index, value) {
if (value.length > 1) {
return;
}
this.otpValues[index] = value;
if (value.length === 1 && index < this.otpValues.length - 1) {
this.$refs.otpFields[index + 1].focus();
}
},
handleBackspace(index) {
if (this.otpValues[index] !== '') {
this.otpValues[index] = '';
} else if (index > 0) {
this.$refs.otpFields[index - 1].focus();
}
},
},
});
app.mount('#app');
</script>
Output of Vue JS OTP Input Example
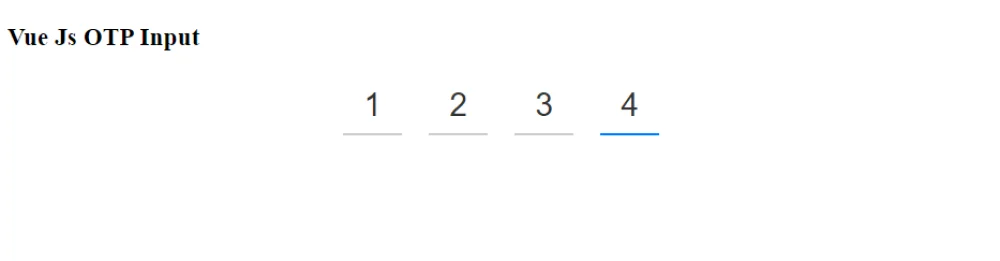