Vue JS Push to Array Function: In Vue.js, the push() method can be used to add new elements to an existing array. When using push(), the new element will be appended to the end of the array and the length of the array will be modified accordingly. This method is particularly useful when working with dynamic data, such as user input or data fetched from an API, where new elements need to be added to an array dynamically. By using push(), you can add new elements to the array without needing to re-render the entire component, making it an efficient way to handle changes in data.
What is the syntax for adding an element to an array in Vue.js using the native push() method, and how does it work?
The code demonstrates how to use Vue.js to add an element to an array using the push method. When the button is clicked, the function adds a new element ‘Africa’ to the countries array. The list of countries is then displayed using a loop that iterates over the countries array and displays each country in a list item. This approach makes it easy to dynamically add elements to an array and update the UI accordingly without having to manually manipulate the DOM. By using Vue.js, developers can create reactive and dynamic web applications that can handle changes in data efficiently.
Vue Js insert an element into an array Example
<div id="app">
<button @click="myFunction">Add country </button>
<p>Add new country in array</p>
<ul v-for='(country,index) in countries' :key='index'>
<li>{{country}}</li>
</ul>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
countries:['India','England','America','Australia','China','Russia'],
}
},
methods:{
myFunction(){
this.results = this.countries.push('Africa');
},
}
}).mount('#app')
</script>
Output of Vue Js Push to Array Method
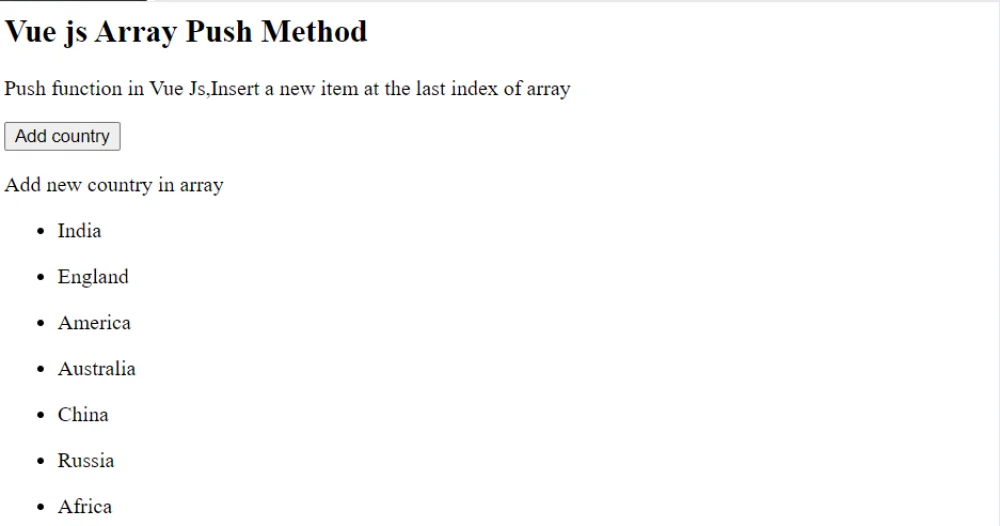
What method can you use to insert two items into an array in Vue.js?
In Vue.js, to add an element or multiple elements to an array, the push() method can be used. This method extends the array by one or more elements and returns the new length of the array. By passing the element or elements as arguments to the push() function, the element or elements will be appended to the end of the array. This approach is particularly useful when dealing with dynamic data or user input, where new elements need to be added to an array dynamically
Vue Js Add two Elements in Array | Example
<div id="app">
<button @click="myFunction">Insert new Number</button>
<p>Numbers : {{numbers}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
numbers:['1','2','3','4','5','6'],
}
},
methods:{
myFunction(){
this.results = this.numbers.push('7','8');
},
}
}).mount('#app')
</script>
Output of above example

What is the syntax for adding a new property to an object in an array of objects in Vue.js
This JavaScript code creates an instance of a Vue.js application that uses the data property to define an array of two website objects, each containing information about a site’s serial number, name, and URL.
The methods property defines a myFunction method that adds a new website object to the sites array with a sno of 3, siteName of ‘Font Awesome Icons’, and siteUrl of ‘https://fontawesomeicons.com/‘ when the button is clicked.
This approach allows developers to add or modify elements of an array dynamically without the need to manipulate the DOM directly. With Vue.js, the app can efficiently update the UI when changes are made to the data.
Vue js Add New Property to Array of Object | Example
<div id="app">
<button @click="myFunction">Add </button>
<table>
<thead>
<tr>
<th>S.no</th>
<th>Site Name</th>
<th>Site Url</th>
</tr>
</thead>
<tbody>
<tr v-for='(site,index) in sites' :key='index'>
<td>{{site.sno}}</td>
<td>{{site.siteName}}</td>
<td><a :href='site.siteUrl' target="_blank">{{site.siteUrl}}</a></td>
</tr>
</tbody>
</table>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
sites:[
{
sno : 1,
siteName : 'Sarkari Naukri Exams',
siteUrl : 'https://www.sarkarinaukriexams.com/'
},
{
sno : 2,
siteName: 'Tutorials Plane',
siteUrl:'https://www.tutorialsplane.com/'
}
],
}
},
methods:{
myFunction(){
this.results = this.sites.push({sno:3,siteName:'Font Awesome Icons',siteUrl:'https://fontawesomeicons.com/'});
},
}
}).mount('#app')
</script>
Output of Push to Array of Object
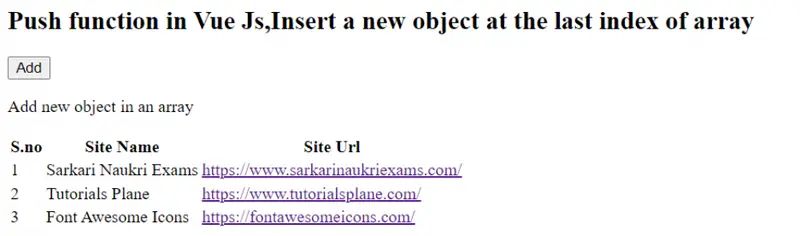