Vue JS Push to Ref Array: In Vue.js, reactive arrays are arrays that are tracked by the Vue.js framework, meaning that any changes made to them are automatically detected and updated in the user interface (UI). The push
method is used to add an item to the end of a reactive array, and Vue.js will detect this change and automatically update the UI. This is useful because it allows developers to easily manipulate the data of their application without needing to manually update the UI. By using reactive arrays, developers can create dynamic and responsive user interfaces with ease, making Vue.js a powerful tool for building complex web applications.
What is a reactive array in Vue.js and how does the push
method work in pushing an item to a reactive array?
This code creates a Vue application that uses the ref
function from Vue to create a reactive array called myArray
containing two items. There is also a newItem
variable initialized with an empty string.
The addItem
function checks if newItem
is not empty and if it is not, it pushes the value of newItem
to the end of myArray
using the push
method. It then sets the newItem
value back to an empty string.
The setup
function returns an object with myArray
, newItem
, and addItem
properties. These properties are used in the template of the Vue application to display the myArray
items and allow the user to add new items by typing in the input field and clicking the “Add” button.
Vue Js Push to Reactive Array Example
<div id="app">
<form @submit.prevent="addItem">
<input type="text" v-model="newItem" placeholder="Add new item">
<button type="submit">Add</button>
</form>
<ul>
<li v-for="item in myArray" :key="item">{{ item }}</li>
</ul>
</div>
<script>
const { ref } = Vue;
const app = Vue.createApp({
setup() {
const myArray = ref(['item 1', 'item 2']);
const newItem = ref('');
const addItem = () => {
if (newItem.value !== '') {
myArray.value.push(newItem.value);
newItem.value = '';
}
};
return {
myArray,
newItem,
addItem,
};
},
});
app.mount('#app');
</script>
Output of Vue js push to ref Array
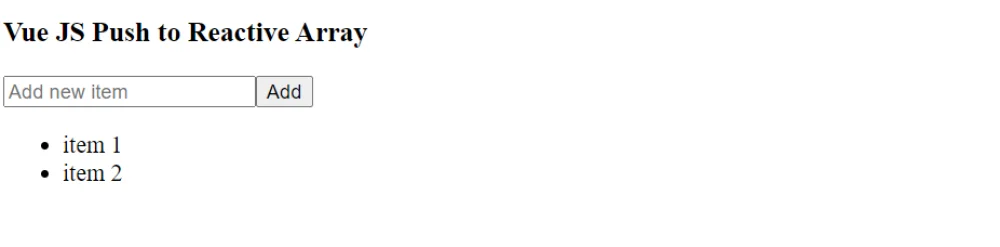