Vue Js Reload/Refresh Image with Same url onclick: In Vue.js, to reload an image URL on a click event, a unique query parameter can be added to the URL each time the image is clicked. This can be achieved by appending a timestamp or a random number to the end of the URL using a computed property or a method. By doing so, the browser is forced to fetch the image from the server, even if the URL is the same, thus ensuring that the latest version of the image is displayed. This technique can be useful in scenarios where the image content is updated frequently and needs to be refreshed without the user having to manually refresh the page.
How can I reload or refresh an image in Vue js with the same URL when a user clicks on it?
To reload/refresh an image with the same URL on click in Vue.js, you can create a method that updates the URL with a new timestamp, forcing the browser to reload the image. Here is an example implementation based on your code:
Vue Js Reload/Refresh Image with Same url onclick Example
<div id="app">
<img :src="imageUrl" @click="reloadImage">
<small>Url: {{imageUrl}}</small>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
imageUrl: "https://pixabay.com/get/g6a6aba109c8c9fee289f57c049bd8b2d7beda5c7a3258f810cfa0bc03d5295f6d05fb2dbfb2efa71cbba67e95f400c38ac9530581524a73ff1d8f64fdeb7e197_640.jpg",
};
},
methods: {
reloadImage() {
const timestamp = new Date().getTime();
this.imageUrl = `https://pixabay.com/get/g6a6aba109c8c9fee289f57c049bd8b2d7beda5c7a3258f810cfa0bc03d5295f6d05fb2dbfb2efa71cbba67e95f400c38ac9530581524a73ff1d8f64fdeb7e197_640.jpg?${timestamp}`;
}
}
})
</script>
Output of Vue Js Reload/Refresh Image with Same url onclick
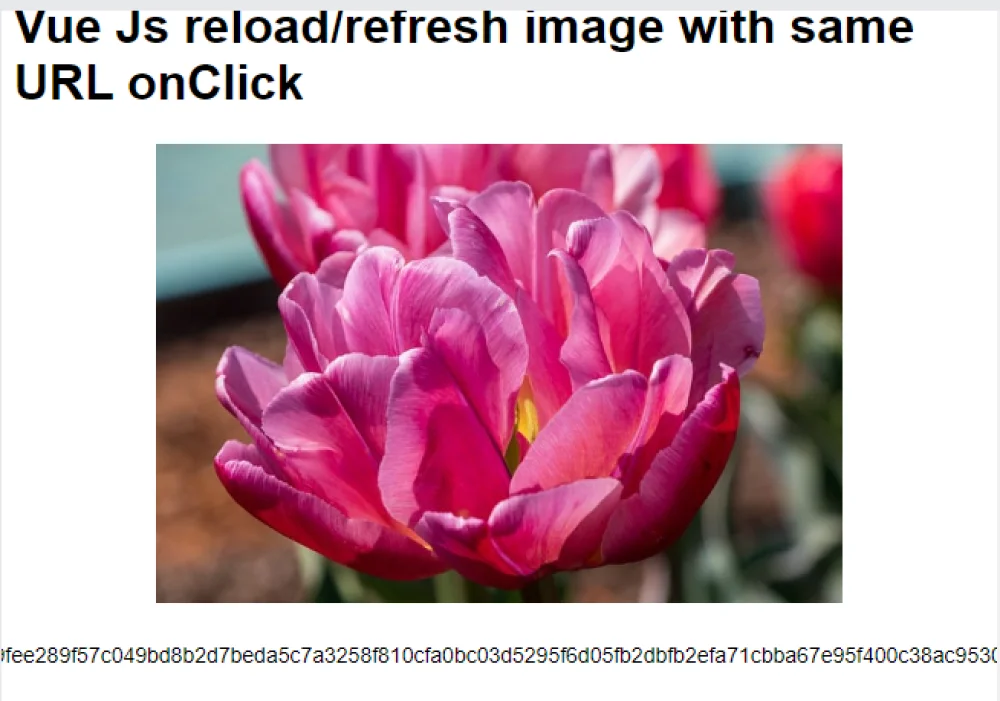