Vue Js Remove Empty or null Object from JSON:In Vue.js, you can remove empty or null objects from a JSON array using the filter
method. The filter
method creates a new array with all elements that pass the test implemented by the provided function. To remove empty or null objects, you can use a callback function that checks if the object is not null or empty.
How can you remove empty or null objects from a JSON data using Vue js?
This code defines a Vue.js application that has a data
property named json
, which is an array of objects. Some of these objects have empty properties, such as {}
. The goal is to filter out these empty objects from the array.
To do this, a computed property named filteredJson
is defined. This property uses the filter()
method of the json
array to create a new array that only includes objects with a non-zero number of keys. The Object.keys(obj).length !== 0
condition is used to determine whether an object has at least one property.
Vue Js Remove Empty or null Object from JSON Example
<script type="module" >
const app = new Vue({
el: "#app",
data() {
return {
json: [
{ name: "John", age: 30 },
{},
{ name: "Jane", age: 25 },
{},
{ name: "Bob", age: 40 }
]
};
},
computed: {
filteredJson() {
return this.json.filter(obj => {
return Object.keys(obj).length !== 0;
});
}
}
});
</script>
Output of Vue Js Remove Empty or null Object from JSON
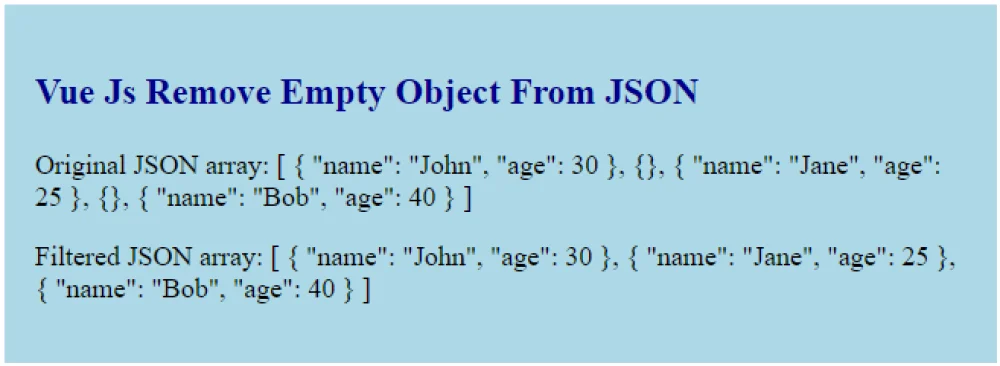