Vue JS Remove Empty or Null Value from JSON:Vue.js is a popular JavaScript framework used for building user interfaces. When working with JSON data in Vue.js, it’s sometimes necessary to remove empty or null values. One way to do this is by using the JSON.stringify()
method, which converts a JavaScript object to a JSON string. By providing a replacer function as the second argument to JSON.stringify()
, we can customize the stringification process to remove empty or null values from the resulting JSON string. The replacer function can examine each value in the object and return undefined
for values that should be removed. The resulting JSON string can then be parsed back into a JavaScript object using JSON.parse()
How can empty or null values be removed from a JSON object in Vue js?
The Below code filters out null values and empty strings from originalObjs using map(), JSON.stringify(), and JSON.parse(). It passes a function as the second argument to JSON.stringify() to remove null values and empty strings. The resulting string is parsed back to a JSON object using JSON.parse(). The filtered objects are stored in the filteredObjs property. This approach creates new objects with the filtered data, leaving the original objects unmodified.
Vue JS Remove Empty or Null Value from JSON Example
<script type="module" >
const app = new Vue({
el: "#app",
data() {
return {
originalObjs: [
{
name: 'John',
age: null,
address: {
street: '123 Main St',
city: '',
state: 'CA'
}
},
{
name: 'Jane',
age: 25,
address: {
street: '456 Oak Ave',
city: 'New York',
state: null
}
}
],
filteredObjs: null
};
},
mounted() {
// Filter each object in originalObjs array
this.filteredObjs = this.originalObjs.map(obj => {
// Convert object to string and remove null values and empty strings
const filteredJSON = JSON.stringify(obj, (key, value) => {
return (value === null || value === '') ? undefined : value;
});
// Convert string back to JSON object
return JSON.parse(filteredJSON);
});
}
});
</script>
Output of Vue JS Remove Empty or Null Value from JSON
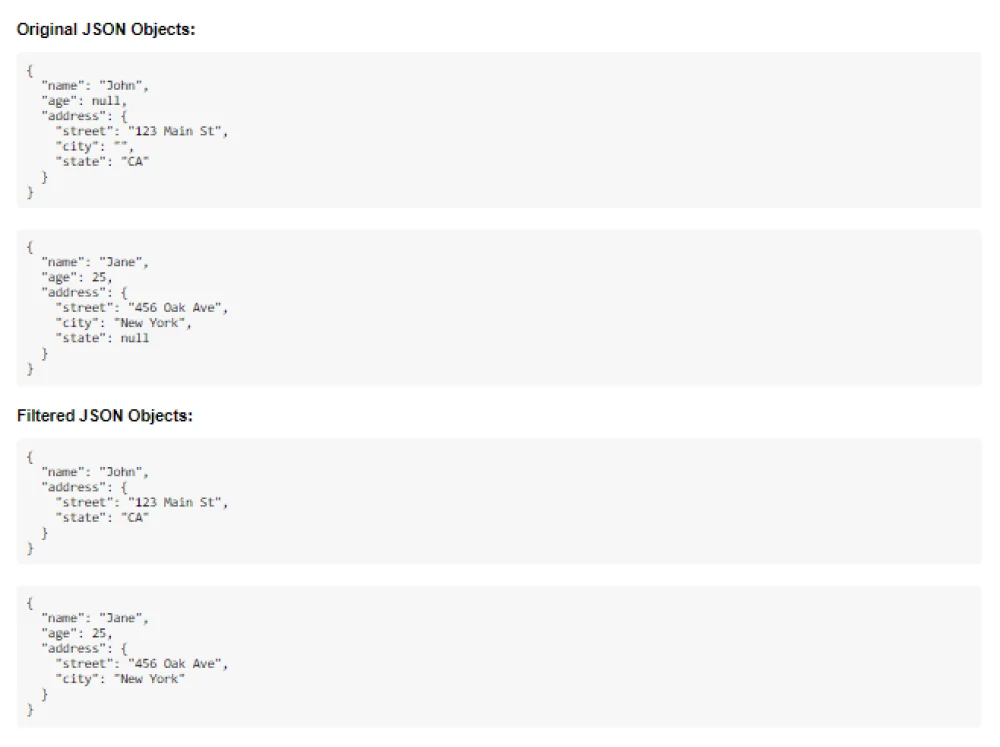