Vue Js Remove Last Comma of String: Vue Js is a JavaScript framework used for building user interfaces. If you need to remove the last comma from a string in Vue Js, you have several options.
First, you can use the String.prototype.slice() method to remove the last comma by specifying the start and end positions of the string. Second, you can use the String.prototype.substring() method, which is similar to slice() but uses the start and end positions differently. Third, you can use the String.prototype.substr() method to remove the last comma by specifying the starting position and the length of the substring. Finally, you can use regular expressions to match the last comma in the string and remove it using the String.prototype.replace() method.
All of these methods achieve the same goal of removing the last comma from a string in Vue Js, but each has a slightly different syntax and approach. Choose the method that best fits your specific use case and coding style.
Here is an example of how to remove the last comma of a string in Vue.js
In the mounted() hook, it checks if “string” ends with a comma using the endsWith() method. If it does, it removes the last comma using the slice() method and assigns the result to the “result” property.
Overall, this code is checking if the “string” property ends with a comma and removing it if it does.
Vue Js Remove last Comma of String using String.prototype.slice() Method Example
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
string: "React,Vue,Angular,",
result: ''
}
},
mounted() {
if (this.string.endsWith(',')) {
// If it does, remove the last comma using slice
this.result = this.string.slice(0, -1)
}
}
})
</script>
Output of Vue Js Remove last Comma of String
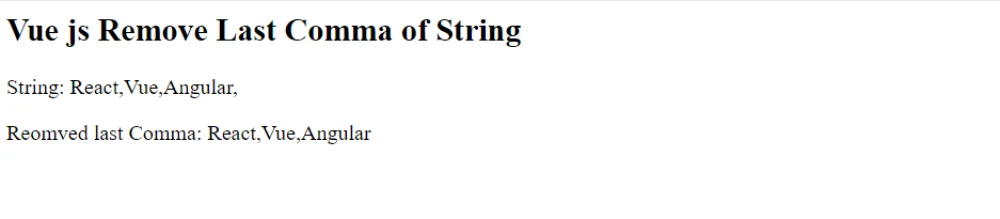
How can you use String.prototype.substring() to remove the last comma from a string in Vue.js?
The “mounted” lifecycle hook runs after the application is mounted to the DOM, and it sets “result” to “string” with the last comma removed using the “substring” method.
Method 2: Using String.prototype.substring()
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
string: "example string,",
result: ''
}
},
mounted() {
this.result = this.string.substring(0, this.string.length - 1); // remove last comma
}
})
</script>
Using String.prototype.substr() to Remove the Last Comma in Vue.js
In Vue.js, String.prototype.substr() can be used to remove the last comma from a string. This method takes two arguments: the starting index and the number of characters to extract. By providing the appropriate arguments, the last comma can be extracted and removed from the string.
Method 3: Using String.prototype.substr()
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
string: "This is a string with a comma at the end,",
result: ''
}
},
mounted() {
this.result = this.string.substr(0, this.string.length - 1); // remove last character
}
})
</script>
How can I remove the last comma from a string in Vue.js using regular expressions?
To remove the last comma from a string in Vue.js using regular expressions, you can use the replace()
method on the string with a regular expression pattern that matches the last comma in the string.
Method 4: Using Regular Expressions
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
string: "Font Awesome Icons,",
result: ''
}
},
mounted() {
this.result = this.string.replace(/,$/, ""); // remove last character
}
})
</script>
Output of above example
