Vue Js Replace Space with Underscore:In Vue.js, to replace spaces with underscores in a string, you can use the replace
method of a string and provide it with a regular expression to match spaces and replace them with an underscore.
How can we replace spaces with underscores in Vue js?
The Below code is a basic Vue.js application that replaces spaces with underscores in a user input string. Here’s a breakdown of how it works:
- The HTML code defines a label, an input field, and a paragraph element that will display the modified string.
- The Vue.js application is defined using the “const app” variable, and is bound to the HTML element with the “id” of “app”.
- The “data” object in the Vue.js application contains a single property, “userString”, which is initialized with the value “Welcome to Font awesome Icons”.
- The “computed” property in the Vue.js application defines a method called “modifiedString”. This method uses the “replace()” function with a regular expression to replace all occurrences of one or more spaces with an underscore in the “userString” value. The modified string is then returned by the method.
Vue Js Replace Space with Underscore Example
<div id="app">
<label for="userInput">Enter a string:</label>
<input id="userInput" v-model="userString">
<p>{{ modifiedString }}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
userString: "Welcome to Font awesome Icons",
};
},
computed: {
modifiedString() {
return this.userString.replace(/\s+/g, "_");
},
},
})
</script>
Output of Vue Js Replace Space with Underscore
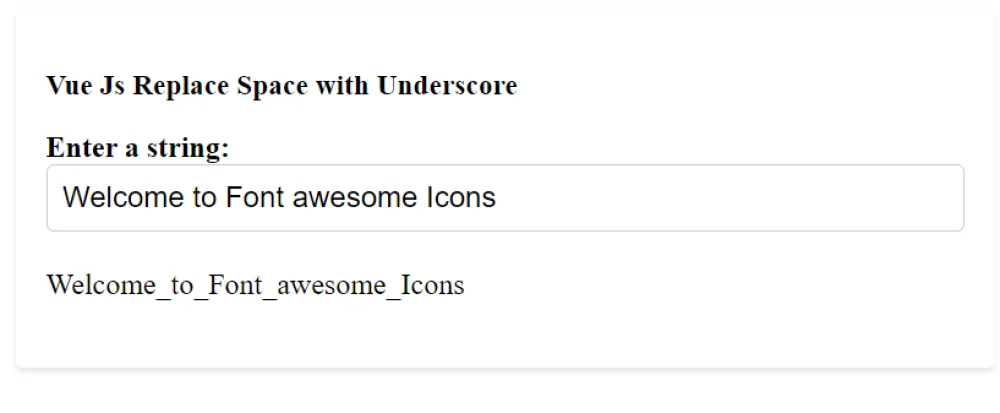