Vue Js Scroll to Element by Id:Vue.js provides a straightforward way to scroll to an element by its ID using the scrollIntoView()
method. We can retrieve the reference to the desired element using the getElementById()
method and then call scrollIntoView()
on that element. This method scrolls the element into view, aligning it to the top of the viewport by default. We can pass an object as an argument to scrollIntoView()
to specify additional options, such as changing the alignment or adding smooth scrolling behavior. By utilizing scrollIntoView()
, we can easily navigate to the desired location in the document without the need for manual scrolling
How to smoothly Vue Js scroll to an element by ID?
This is a Vue.js example that demonstrates how to create a list of elements and scroll to a particular element on clicking a button. In this example, the v-for
directive is used to loop through the elements
array and display each element in a div with a unique id
. The same id
is also assigned to the corresponding button’s data-id
attribute.
When a button is clicked, the scrollToElement
method is called, which takes the id
of the corresponding element as an argument. The method then uses the getElementById
method to get a reference to the element, and the scrollIntoView
method is used to scroll the element into view with a smooth behavior.
This example demonstrates how Vue.js can be used to create dynamic interfaces that respond to user interactions, allowing you to create rich and engaging user experiences.
Vue Js Scroll to Element by Id Example
<div id="app">
<button v-for="(element, index) in elements" :key="index" @click="scrollToElement(element.id)">{{ element.name
}}</button>
<div v-for="(element, index) in elements" :key="index" :id="element.id" class="element">
<h2>{{ element.name }}</h2>
<p>{{ element.description }}</p>
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
elements: [
{ id: 'element1', name: 'Element 1', description: 'This is the first element' },
{ id: 'element2', name: 'Element 2', description: 'This is the second element' },
{ id: 'element3', name: 'Element 3', description: 'This is the third element' },
]
};
},
methods: {
scrollToElement(id) {
const container = document.getElementById(id);
container.scrollIntoView({ behavior: 'smooth' });
}
},
});
</script>
Outrput of Vue Js Scroll to Element by Id
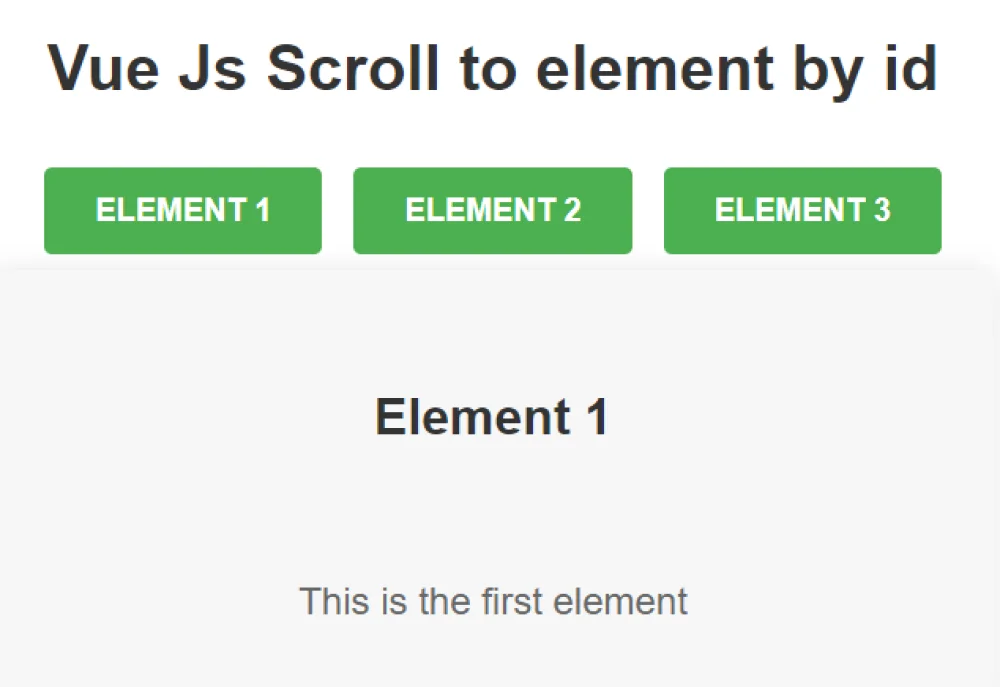