Vue Js Select On Change Update Value –: In this tutorial, we will learn how to use Vuejs to create a select dropdown element and update its value when the user makes a selection. We will implement an event handler that listens for changes to the select element and updates a data property in the Vue instance accordingly. This way, we can reactively update other parts of our application based on the user’s selection in the dropdown.
How can I implement a Vuejs select element that updates a value when its option is changed
This Vuejs code creates a custom select element with options for different fruits. When the user selects a fruit, the “Selected Fruit” paragraph dynamically updates to show the chosen fruit. The v-model
directive binds the selected fruit to the selectedFruit
data property. A watch function is used to track changes in the selectedFruit
property, allowing you to perform additional logic when the selection changes. In this example, a message is logged to the console with the newly selected fruit’s value.
Vue js Select On Change Update Value Example
<div id="app">
<div class="custom-select-wrapper">
<h3>Vue js Select On Change Update Value</h3>
<select class="custom-select" v-model="selectedFruit">
<option
v-for="fruit in fruits"
:key="fruit.value"
:value="fruit.value"
>
{{ fruit.label }}
</option>
</select>
<p>Selected Fruit: {{ selectedFruit }}</p>
</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
selectedFruit: null,
fruits: [
{ value: "apple", label: "Apple" },
{ value: "banana", label: "Banana" },
{ value: "orange", label: "Orange" },
],
};
},
watch: {
selectedFruit(newValue) {
// You can perform any logic here when the fruit selection changes
console.log("Selected fruit changed:", newValue);
},
},
});
app.mount("#app");
</script>
Output of Vue js Select On Change Update Value
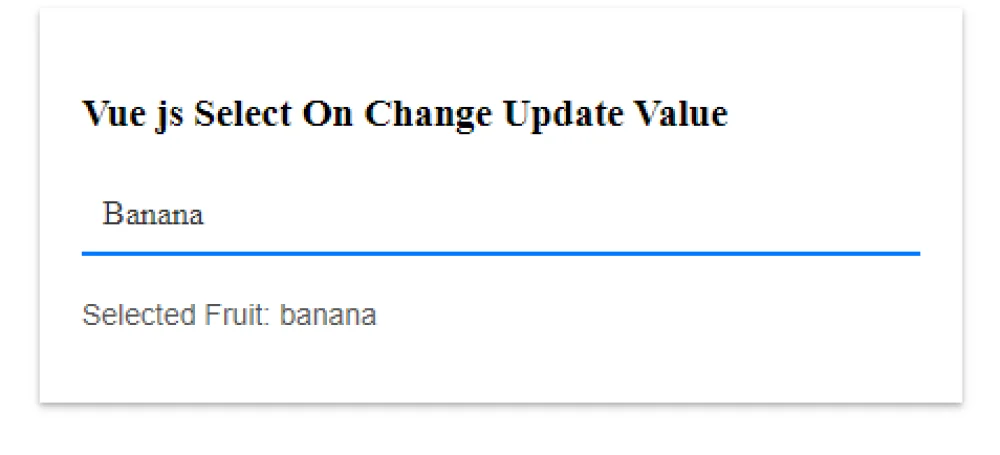