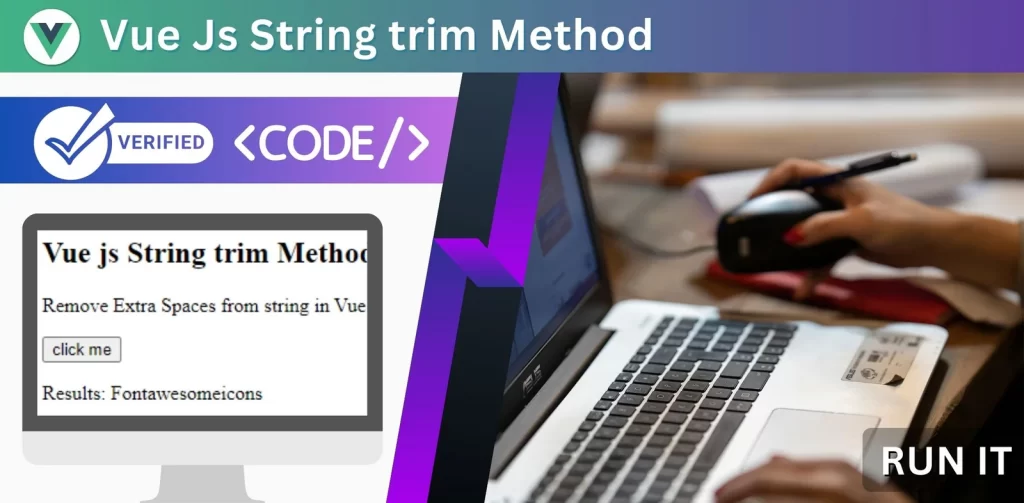
Vue Js String Trim Method: Vue.js String trim is a function used to remove whitespace characters from the beginning and end of a given string . The trim() method removes whitespace from both sides of a string. This method can be useful for cleaning up user input and removing unnecessary whitespace from strings that may have been created through concatenation Here in this tutorial, we will learn how to use the native Javascript trim() function with Vue JS. Examples are given below
Using Vue.js, how to eliminate spaces from a string?
Removing spaces from a string in Vue.js can be done with the trim() function, which takes no arguments and returns a new string with whitespace removed from the beginning and end of the original string
Vue Js remove space between string
<div id="app">
<button @click="myFunction">click me</button>
<p>Results: {{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
text:" Fontawesomeicons ",
results:''
}
},
methods:{
myFunction(){
this.results = this.text.trim();
},
}
}).mount('#app')
</script>
Output of above example
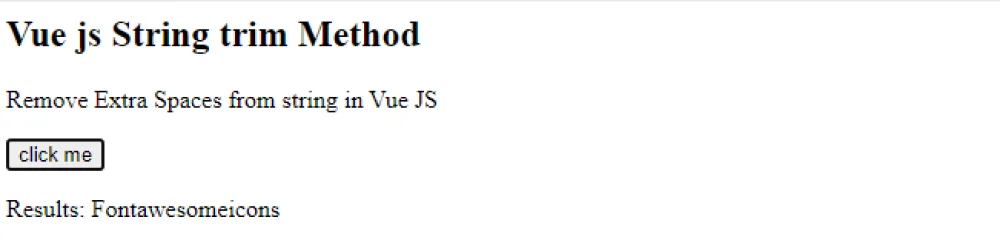
How to use a regular expression in Vue JS to remove spaces with replace()?
The replace() method in Vue JS can be used to remove spaces from strings with a regular expression
Vue JS Remove white space with replace Method | Example
<div id="app">
<button @click="myFunction">click me</button>
<p>Return String: {{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
string:" Fontawesomeicons ",
results:''
}
},
methods:{
myFunction(){
this.results = this.string.replace(/^\s+|\s+$/gm,'');
},
}
}).mount('#app')
</script>
Output of above example
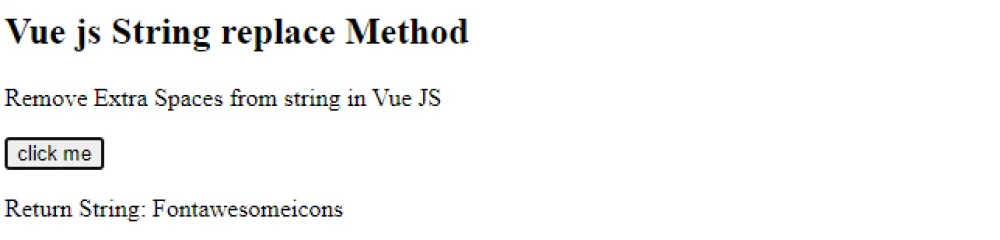