Vue Js Expand Collapse Table: A Vue.js expand-collapse table is a table that allows users to view and hide table rows as needed. It typically includes a clickable icon or button that expands or collapses the row, revealing or hiding additional information. This functionality can be achieved using Vue.js directives such as v-if and v-show, which allow developers to conditionally render elements based on the state of the application. By using these directives in conjunction with event listeners and state management tools such as Vuex, developers can create dynamic and responsive tables that enhance the user experience by providing more control and flexibility over the displayed data.
How can I implement expand and collapse functionality in a table using Vue.js?
The code is a Vue.js component that displays a table of user data. Each row in the table represents a user and displays their name, email, and a button to toggle the display of additional details.
The user data is stored in an array of objects, where each object represents a user and contains properties for their name, email, details, and a Boolean value indicating whether or not to show the details.
The table is rendered using a v-for
directive to loop through the users
array and display a row for each user. The toggleDetails
method is called when the button is clicked and toggles the showDetails
property for the corresponding user object.
The additional details are displayed in a separate row below the user’s row, using the v-if
directive to conditionally render the row based on the value of showDetails
. The colspan
attribute is used to span the entire row.
Vue Js Expand Collapse Table Example
<div id="app">
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Details</th>
</tr>
</thead>
<tbody v-for="(user, index) in users" :key="index">
<tr>
<td>{{ user.name }}</td>
<td>{{ user.email }}</td>
<td>
<button @click="toggleDetails(index)">
{{ user.showDetails ? 'Hide Details' : 'Show Details' }}
</button>
</td>
</tr>
<tr>
<td colspan="3" v-if="user.showDetails"
:class="{'open-details': user.showDetails, 'close-details': !user.showDetails}">
{{ user.details }}
</td>
</tr>
</tbody>
</table>
</div>
<script>
new Vue({
el: '#app',
data() {
return {
users: [
{
name: 'John Doe',
email: 'john@example.com',
details: 'John Doe is a web developer from San Francisco',
showDetails: false
},
{
name: 'Jane Smith',
email: 'jane@example.com',
details: 'Jane Smith is a graphic designer from New York',
showDetails: false
},
{
name: 'Andrew',
email: 'andrew@example.com',
details: 'Andrew is a Backend Developer from USA',
showDetails: false
},
{
name: 'James',
email: 'james@example.com',
details: 'James is a fullstack developer from England',
showDetails: false
}
]
};
},
methods: {
toggleDetails(index) {
this.users[index].showDetails = !this.users[index].showDetails;
}
}
});
</script>
Output of Vue Js Expand Collapse Table
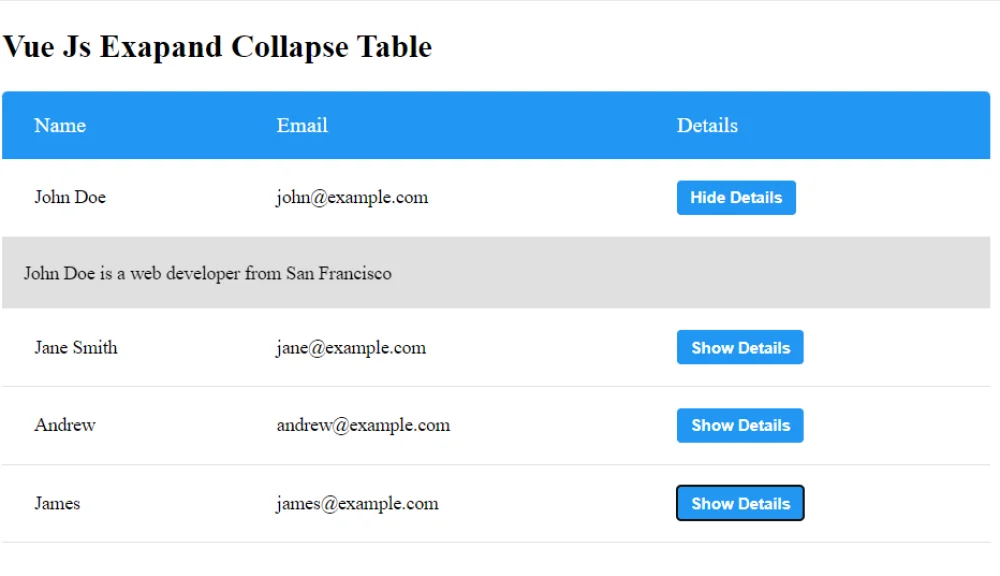