Vue Js Username And Password Validation:Vue.js is a popular front-end JavaScript framework that allows you to easily implement complex UI interactions. When it comes to username and password validation, Vue.js provides a simple and effective way to handle the input and check if the values match certain criteria. To implement this, you can create a form component with two input fields for username and password, and use Vue.js v-model directive to bind the input values to the component’s data. You can then add a validation method that checks if the input values meet your criteria, such as minimum length, presence of special characters or capital letters, and display appropriate error messages if the validation fails. This ensures that users provide valid login credentials before accessing your application.
How to validate username and password in Vue.js?
This is a Vue.js script for validating a username and password input form.
The script defines a Vue instance with data properties for the username, password, and validation message, as well as methods for submitting the form and checking the validity of the input.
https://googleads.g.doubleclick.net/pagead/ads?gdpr=0&client=ca-pub-8816212884634075&output=html&h=280&adk=3469078437&adf=311551810&w=1155&abgtt=11&fwrn=4&fwrnh=100&lmt=1730558487&num_ads=1&rafmt=1&armr=3&sem=mc&pwprc=9568628027&ad_type=text_image&format=1155×280&url=https%3A%2F%2Ffontawesomeicons.com%2Ffa%2Fvue-js-username-and-password-validation&fwr=0&pra=3&rh=200&rw=1155&rpe=1&resp_fmts=3&wgl=1&fa=27&uach=WyJXaW5kb3dzIiwiMTAuMC4wIiwieDg2IiwiIiwiMTMwLjAuNjcyMy43MCIsbnVsbCwwLG51bGwsIjY0IixbWyJDaHJvbWl1bSIsIjEzMC4wLjY3MjMuNzAiXSxbIkdvb2dsZSBDaHJvbWUiLCIxMzAuMC42NzIzLjcwIl0sWyJOb3Q_QV9CcmFuZCIsIjk5LjAuMC4wIl1dLDBd&dt=1730558487397&bpp=1&bdt=894&idt=-M&shv=r20241030&mjsv=m202410290101&ptt=9&saldr=aa&abxe=1&cookie=ID%3D52d462117c90aa4e%3AT%3D1716985591%3ART%3D1730558484%3AS%3DALNI_MaymvdUQ3-gCrExazpHpkPzpW280w&gpic=UID%3D00000e33bc62f602%3AT%3D1716985591%3ART%3D1730558484%3AS%3DALNI_Mbw4BXutBGHB1jq4hjK85WOXyq3tQ&eo_id_str=ID%3D27c2104b1c6398ee%3AT%3D1716985591%3ART%3D1730558484%3AS%3DAA-Afjbr3iJZTiXPAGIkj6QDzEHX&prev_fmts=1135×280%2C1135x200%2C870x120%2C1135x280%2C214x600%2C214x600%2C0x0%2C0x0&nras=3&correlator=490177260357&frm=20&pv=1&u_tz=420&u_his=23&u_h=1080&u_w=1920&u_ah=1040&u_aw=1920&u_cd=24&u_sd=1&dmc=8&adx=317&ady=1030&biw=1903&bih=953&scr_x=0&scr_y=0&eid=44759875%2C44759926%2C31088481%2C31088581%2C95331832%2C95344188%2C31088607%2C95345472%2C95345789%2C95345963%2C95345966&oid=2&psts=AOrYGsnPVUJcdHiT0qMdZP2cz4Rky2BPsCEP4X_U6TX5x5OLFqzSHppEGl2odJl8tTFoeUZ3URwV86kMrP64-ayDrJjD&pvsid=4364340874797652&tmod=824333067&uas=0&nvt=1&ref=https%3A%2F%2Ffontawesomeicons.com%2Ffa%2Fvue-js-password-validation-regex&fc=384&brdim=0%2C0%2C0%2C0%2C1920%2C0%2C1920%2C1040%2C1920%2C953&vis=1&rsz=%7C%7Cs%7C&abl=NS&fu=128&bc=31&bz=1&td=1&tdf=2&psd=W251bGwsbnVsbCxudWxsLDNd&nt=1&ifi=13&uci=a!d&btvi=3&fsb=1&dtd=98
The isValid()
method checks the length of the username and password input against defined minimum and maximum values, and uses a regular expression to check if the password contains at least one letter and one number. If any of these criteria are not met, it returns false.
The checkPasswordStrength()
method checks the strength of the password using a regular expression, and returns a string indicating whether the password is weak, medium, or strong.
The validationMessage()
computed property returns a string with validation messages for any input fields that are not valid, while the passwordStrength()
computed property returns the strength of the password.
Finally, the submitForm()
method checks the validity of the input and either performs the form submission logic or sets a flag to show the validation message if the input is invalid.
Overall, this script provides basic validation for a username and password input form and can be adapted to suit different requirements
Vue Js Username and Password Validation Example
<div id="app">
<form>
<label>
Username:
<input type="text" v-model="username" />
</label>
<label>
Password:
<input type="password" v-model="password" />
<div v-if="password !== ''">
Password strength: {{ passwordStrength }}
</div>
</label>
<button @click.prevent="submitForm">Submit</button>
<p v-if="showValidationMessage">{{ validationMessage }}</p>
</form>
</div>
<script type="module" >
const app = new Vue({
el: "#app",
data() {
return {
username: "",
password: "",
showValidationMessage: false,
minUsernameLength: 3,
maxUsernameLength: 20,
minPasswordLength: 8,
maxPasswordLength: 30,
};
},
methods: {
submitForm() {
if (this.isValid()) {
// Perform form submission logic
} else {
this.showValidationMessage = true;
}
},
isValid() {
if (
this.username.length < this.minUsernameLength ||
this.username.length > this.maxUsernameLength ||
this.password.length < this.minPasswordLength ||
this.password.length > this.maxPasswordLength
) {
return false;
}
// Use regular expressions to check the format of the username and password
// Return false if the format is invalid
if (!/^(?=.*[A-Za-z])(?=.*\d)[A-Za-z\d]{8,}$/.test(this.password)) {
this.validationMessage = "Password must contain at least 1 letter and 1 number";
return false;
}
return true;
},
checkPasswordStrength() {
// Use a password strength library or regular expressions to determine the strength of the password
// Update the passwordStrength computed property with a string that describes the strength of the password
let passwordStrength = "Weak";
if (/(?=.*[A-Z])(?=.*[a-z])(?=.*\d).{8,}/.test(this.password)) {
passwordStrength = "Strong";
} else if (/(?=.*[a-zA-Z])(?=.*\d).{6,}/.test(this.password)) {
passwordStrength = "Medium";
}
return passwordStrength;
},
},
computed: {
validationMessage() {
let message = "";
if (this.username.length < this.minUsernameLength) {
message += `Username must be at least ${this.minUsernameLength} characters long. `;
}
if (this.username.length > this.maxUsernameLength) {
message += `Username must be less than ${this.maxUsernameLength} characters long. `;
}
if (this.password.length < this.minPasswordLength) {
message += `Password must be at least ${this.minPasswordLength} characters long. `;
}
if (this.password.length > this.maxPasswordLength) {
message += `Password must be less than ${this.maxPasswordLength} characters long. `;
}
return message;
},
passwordStrength() {
return this.checkPasswordStrength();
},
},
});
</script>
Output of Vue Js Username and Password Validation
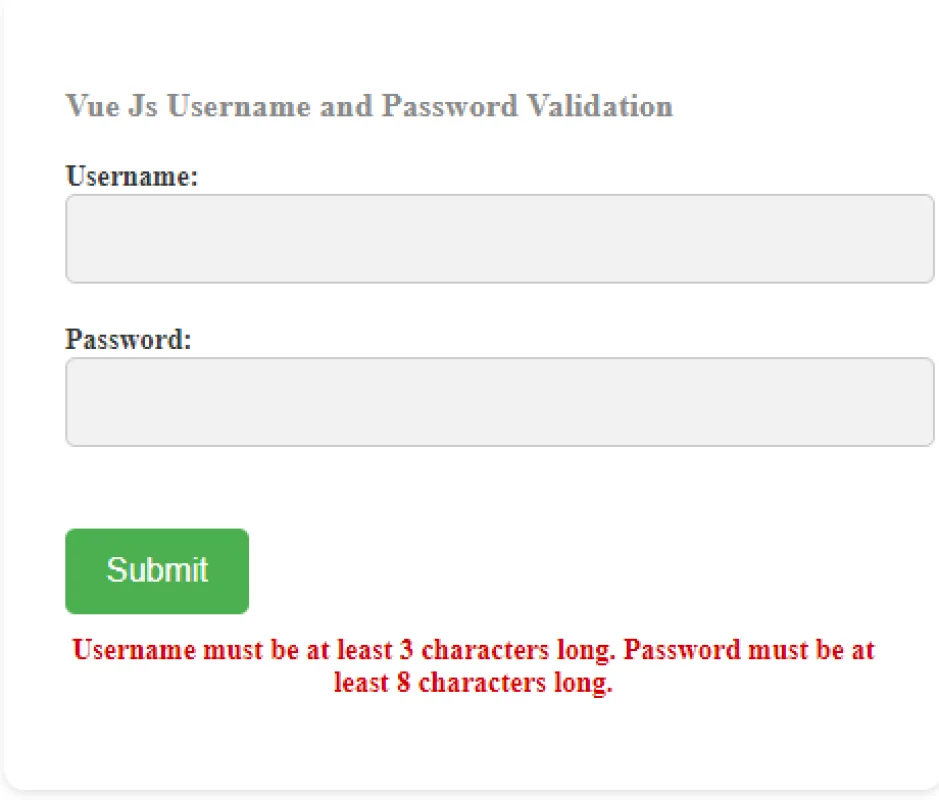