v-if ,v-else – Vue Conditional Rendering: In Vue.js, the v-if
and v-else
directives are used for conditional rendering in templates. v-if
evaluates an expression and renders an element if the expression is true. If it’s false, the element is removed from the DOM. v-else
is used in conjunction with v-if
to specify an alternative element to render if the v-if
condition is false. It helps create dynamic, responsive user interfaces by showing or hiding elements based on data or conditions, making Vue.js a powerful tool for building interactive web applications.
What is the role of the `v-if` directive in Vue js?
The v-if
directive in Vue.js is used to conditionally render HTML elements based on a specified condition. In the given example, the <p>
element with the text “Font Awesome icons” will be rendered inside the <div>
with the id “app” if the showText
data property is true
. If showText
is false
, the <p>
element will not be included in the rendered output. This directive allows dynamic control over what content is displayed in the DOM, making it a fundamental tool for creating responsive and interactive web applications in Vue.js.
Vue Js v-if Directive Example
<div id="app">
<p v-if="showText">Font Awesome icons</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
showText: true
}
},
});
</script>
Output of Vue Js v-if Directive

Explain the purpose and usage of Vue.js v-if
and v-else
directives in conditional rendering?
In Vue.js, the v-if
and v-else
directives facilitate conditional rendering of elements. In your example, when the showText
data property is true
, it displays the “Font Awesome icons” paragraph due to v-if
. Conversely, when showText
is false
, the “condition is false” paragraph appears thanks to v-else
. This dynamic rendering allows developers to show or hide elements based on changing data conditions, enhancing user interfaces by presenting content conditionally without the need for complex JavaScript logic
Vue Js v-if, v-else directive
<div id="app">
<p v-if="showText">Font Awesome icons</p>
<p v-else>condition is false</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
showText: false
}
},
});
</script>
Output of v-else Rendering
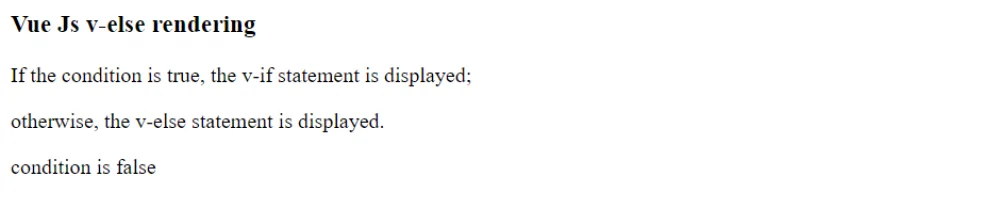
How to Check Multiple Conditions in a Vue Js?
In Vue.js, you can check multiple conditions using the v-if
, v-else-if
, and v-else
directives. In the given example, it checks the value of the name
variable:
- If
name
is ‘David’, it displays the first<p>
element. - If
name
is ‘Andrew’, it displays the second<p>
element. - If none of the above conditions are met, it displays the third
<p>
element.
In this case, since name
is set to ‘Andrew’, the second <p>
element will be displayed. This allows you to conditionally render content based on different values of the name
variable in Vue.js.
Vue Js V-else-if Statement | Example
<div id="app">
<p v-if="name === 'David'">This is statement is display if "name" is 'David' </p>
<p v-else-if="name === 'Andrew'">This is statement is display if "name" is 'Andrew' </p>
<p v-else>This is statement is display if no 'name' is match </p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
name: 'Andrew'
}
},
});
</script>
Output of above example
