In this tutorial, we provide two examples to demonstrate how to use Vue.js setTimeout function for delay or waiting in performance or function, like for 1 second or 5 seconds.
In this example, we will learn how to use setTimeout
with an async function in Vue.js for delaying a function that we want to execute.
Vue Sleep 1 Second
<script type="module">
const app = Vue.createApp({
data() {
return {
buttonColor: 'blue'
};
},
methods: {
async changeColor() {
this.buttonColor = 'red';
await this.sleep(1000);
this.buttonColor = 'blue';
},
sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
}
});
app.mount("#app");
</script>
Output of above example
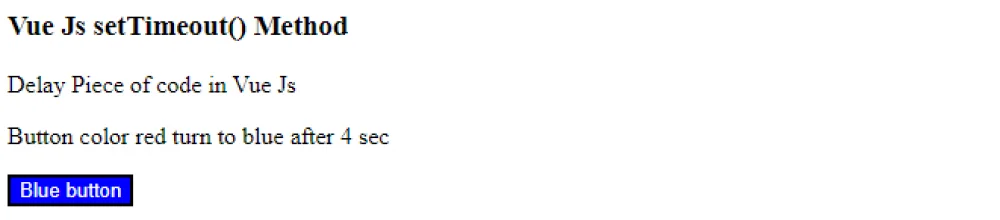
How to Add Delay in Vue Js ?
This is the second example of this tutorial. In the code below, we use methods to implement the ‘settimeout’ function to wait for the result for one second. You can use the ‘tryit’ editor to edit and customize the code.
Vue Delay Function Example
<script type="module">
const app = Vue.createApp({
data() {
return {
result: "",
}
},
methods: {
startDelayedAction() {
this.result = "Loading...";
setTimeout(() => {
this.performAction();
}, 1000);
},
performAction() {
this.result = "Action performed!";
},
},
});
app.mount("#app");
</script>
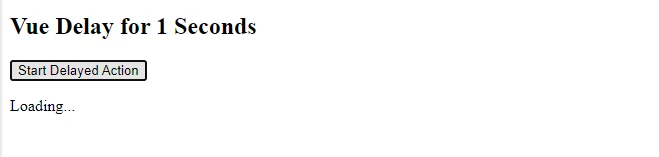