Vue Show Hide Textbox Based On Dropdown Value: To show or hide a textbox based on a dropdown value in Vue, you can use the v-model directive to bind the selected value to a data property. Then, you can use a v-if directive to conditionally render the textbox based on the selected value. For example, you can add a change event handler to the dropdown and update the data property based on the selected value. Then, you can use a computed property to determine whether to show or hide the textbox based on the data property value. The computed property can return a Boolean value that is used in the v-if directive to show or hide the textbox.
How can you implement a feature in Vue that shows or hides a textbox based on the selected value of a dropdown menu?
This code uses Vue to create a form with a dropdown menu to select a fruit, and shows or hides a textbox depending on the selected fruit. It also includes input validation and a button to store the entered data.
The data object contains properties for the selected fruit and the entered values for each textbox.
The methods section includes a function to check if a given value is a number, and a function to store the entered data.
The computed section includes a function to check if the entered data is valid based on the selected fruit.
When the user clicks the “Store Data” button, the entered data is logged to the console and the form is reset
Vue show hide textbox based on dropdown value Example
<div id="app">
<label for="fruit">Select a fruit:</label>
<select id="fruit" v-model="selectedFruit">
<option value="">--Please select--</option>
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="orange">Orange</option>
</select>
<div v-if="selectedFruit === 'apple'">
<label for="appleColor">Enter the color of the apple:</label>
<input id="appleColor" type="text" v-model="appleColor">
<p v-if="appleColor.length === 0" class="error">Please enter a color.</p>
</div>
<div v-if="selectedFruit === 'banana'">
<label for="bananaLength">Enter the length of the banana:</label>
<input id="bananaLength" type="text" v-model="bananaLength">
<p v-if="!isNumber(bananaLength)" class="error">Please enter a number.</p>
</div>
<div v-if="selectedFruit === 'orange'">
<label for="orangeWeight">Enter the weight of the orange:</label>
<input id="orangeWeight" type="text" v-model="orangeWeight">
<p v-if="!isNumber(orangeWeight)" class="error">Please enter a number.</p>
</div>
<button @click="storeData" :disabled="!isValidInput">Store Data</button>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
selectedFruit: '',
appleColor: '',
bananaLength: '',
orangeWeight: '',
}
},
methods: {
isNumber(value) {
return /^\d+$/.test(value);
},
storeData() {
// Do something with the entered data, e.g., send it to a server
console.log({
fruit: this.selectedFruit,
appleColor: this.appleColor,
bananaLength: this.bananaLength,
orangeWeight: this.orangeWeight,
});
// Reset the form
this.selectedFruit = '';
this.appleColor = '';
this.bananaLength = '';
this.orangeWeight = '';
},
},
computed: {
isValidInput() {
if (this.selectedFruit === 'apple') {
return this.appleColor.length > 0;
} else if (this.selectedFruit === 'banana') {
return this.isNumber(this.bananaLength);
} else if (this.selectedFruit === 'orange') {
return this.isNumber(this.orangeWeight);
} else {
return false;
}
},
},
})
app.mount('#app')
</script>
Output of Vue Show Hide Textbox Based On Dropdown Value
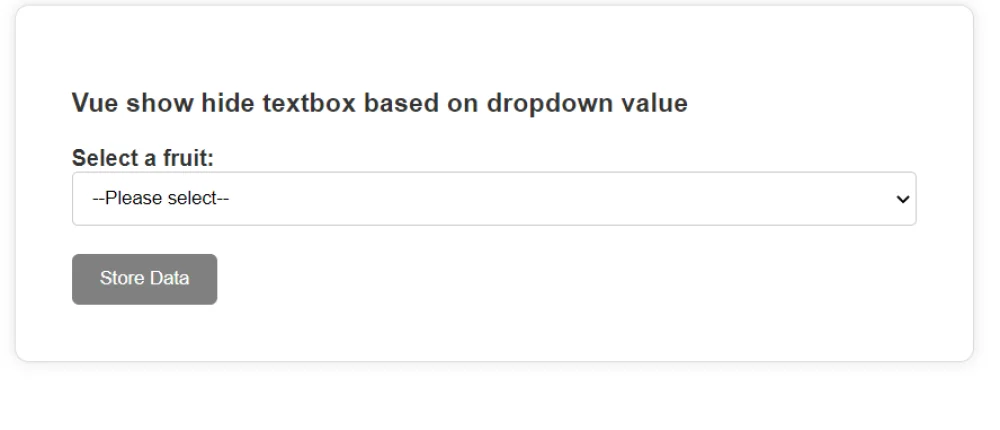