Vue Track Changes in Textarea:In Vue.js, the v-model directive is used to establish two-way data binding between the user input in a textarea element and a corresponding variable in the Vue instance. Whenever the user types something into the textarea, the value of the variable is automatically updated, and vice versa. This allows you to easily track any changes made to the textarea’s contents, and react to those changes in real-time within your application. By using v-model, you can streamline your code and make it more efficient, since you won’t need to write custom event handlers or update functions to keep track of the textarea’s value.
How can Vue be used to track and respond to changes made in a textarea element in real-time?
This code is a Vue application that tracks changes made in a textarea element in real-time and provides undo and redo functionality.
The Vue app has a data object that includes the current value of the textarea, as well as arrays for storing the history of changes and future changes (if undo is used).
The textarea element is bound to the currentValue property using v-model, and the onInput method is called whenever the textarea’s value changes. In onInput, the new value is added to the history array, and the future array is cleared.
The undo and redo methods allow the user to go back and forth between previous and future changes. If the user clicks the undo button, the last value in the history array is popped off and added to the future array, and the currentValue is set to the previous value. If the user clicks the redo button, the last value in the future array is popped off and added to the history array, and the currentValue is set to the next value.
Vue track changes in textarea Example
<div id="app">
<textarea v-model="currentValue" @input="onInput"></textarea>
<button @click="undo">Undo</button>
<button @click="redo">Redo</button>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
currentValue: '',
history: [],
future: []
}
},
methods: {
onInput(event) {
const newValue = event.target.value;
this.future = [];
this.history.push(newValue);
},
undo() {
if (this.history.length > 0) {
const previousValue = this.history.pop();
this.future.push(this.currentValue);
this.currentValue = previousValue;
}
},
redo() {
if (this.future.length > 0) {
const nextValue = this.future.pop();
this.history.push(this.currentValue);
this.currentValue = nextValue;
}
}
}
});app.mount('#app');
</script>
Output of Vue track changes in textarea
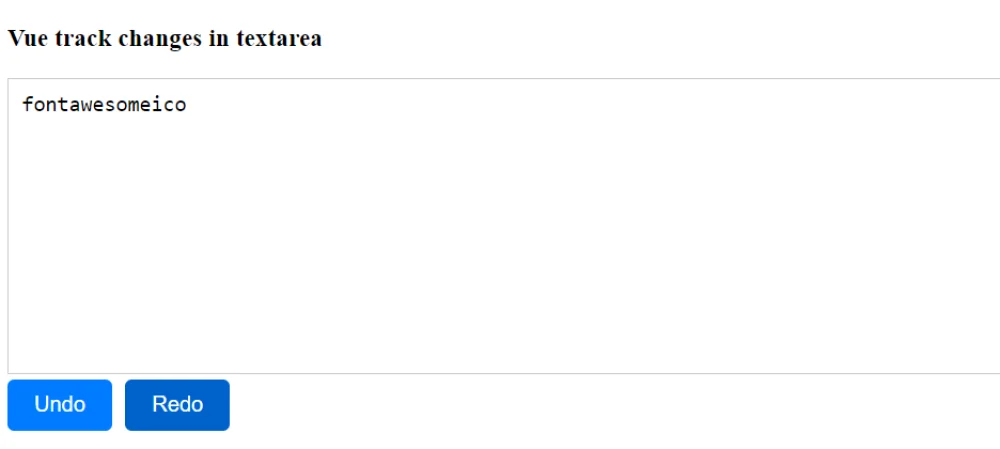