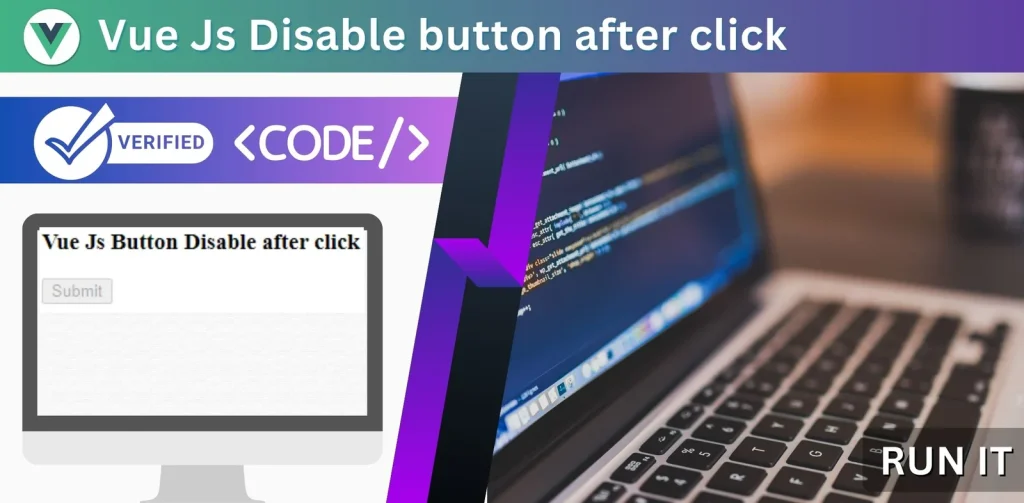
In this tutorial, we will learn how to disable a button on click using Vue 3 for improving user experience and preventing multiple submissions of the same form or action. We will provide two examples using the Composition API and Options API, respectively.
How to Disable Button in Vue Js?
In this example, we cover how to disable a button after a click. We use the Vue Options API to add the disabled attribute to the button and update it accordingly when the user clicks the button
Vue Disable Button After Click (Options Api)
<div id="app">
<button :disabled="isButtonDisabled" @click="disableButton">Submit</button>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
isButtonDisabled: false
}
},
methods: {
disableButton() {
this.isButtonDisabled = true;
}
}
});
</script>
Output of above example

Vue 3 Disable Button on Click (Composition API)
<script type="module">
const {createApp,ref} = Vue;
createApp({
setup() {
const isDisabled = ref(false);
function handleClick() {
// Your logic to determine whether the button should be disabled or not
// For example, disabling the button after it's clicked once
isDisabled.value = true;
// Your other logic...
}
return {
isDisabled,
handleClick
};
}
}).mount("#app");
</script>
Output of Vue Js Disable Button After Click
